友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
21. 合并两个有序链表
将两个升序链表合并为一个新的 升序 链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。
示例 1:
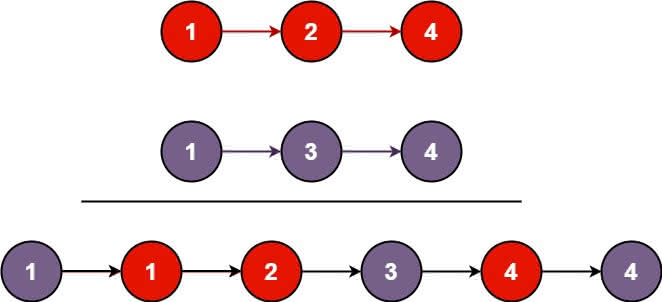
输入:l1 = [1,2,4], l2 = [1,3,4] 输出:[1,1,2,3,4,4]
示例 2:
输入:l1 = [], l2 = [] 输出:[]
示例 3:
输入:l1 = [], l2 = [0] 输出:[0]
提示:
-
两个链表的节点数目范围是
[0, 50]
-
-100 <= Node.val <= 100
-
l1
和l2
均按 非递减顺序 排列
思路分析
最先想到的是迭代。
但是,也可以用递归解决!
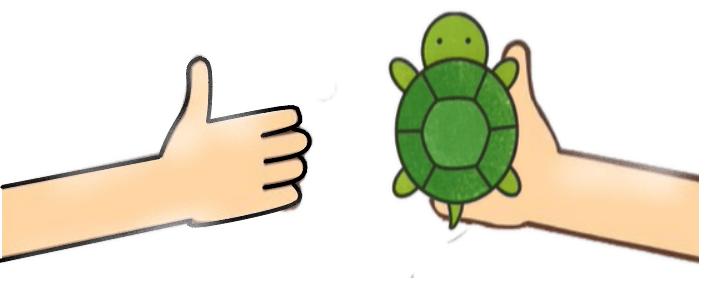
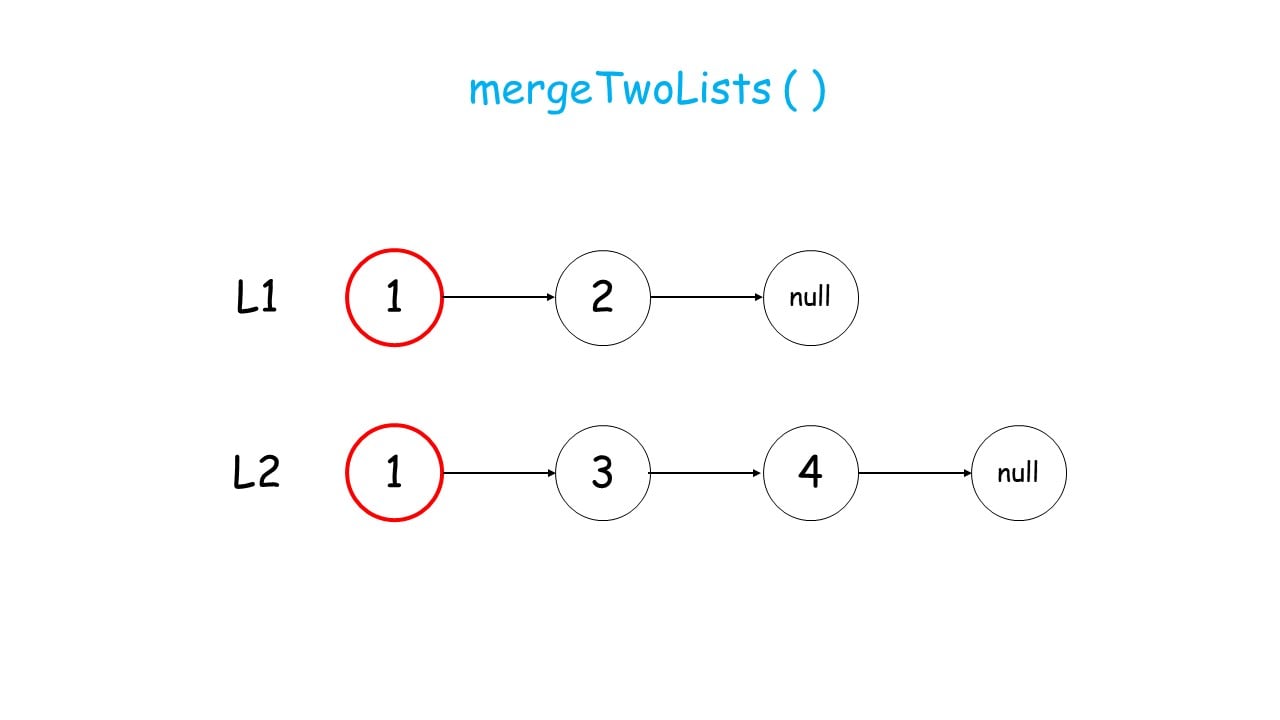
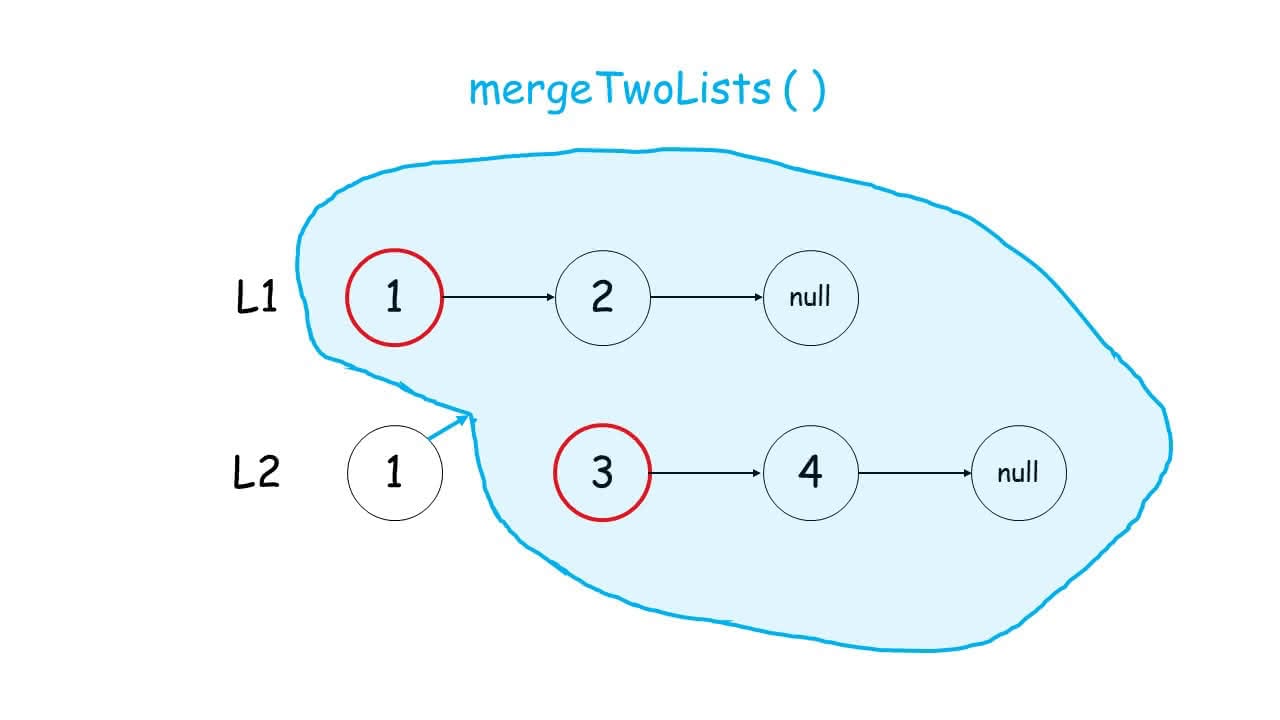
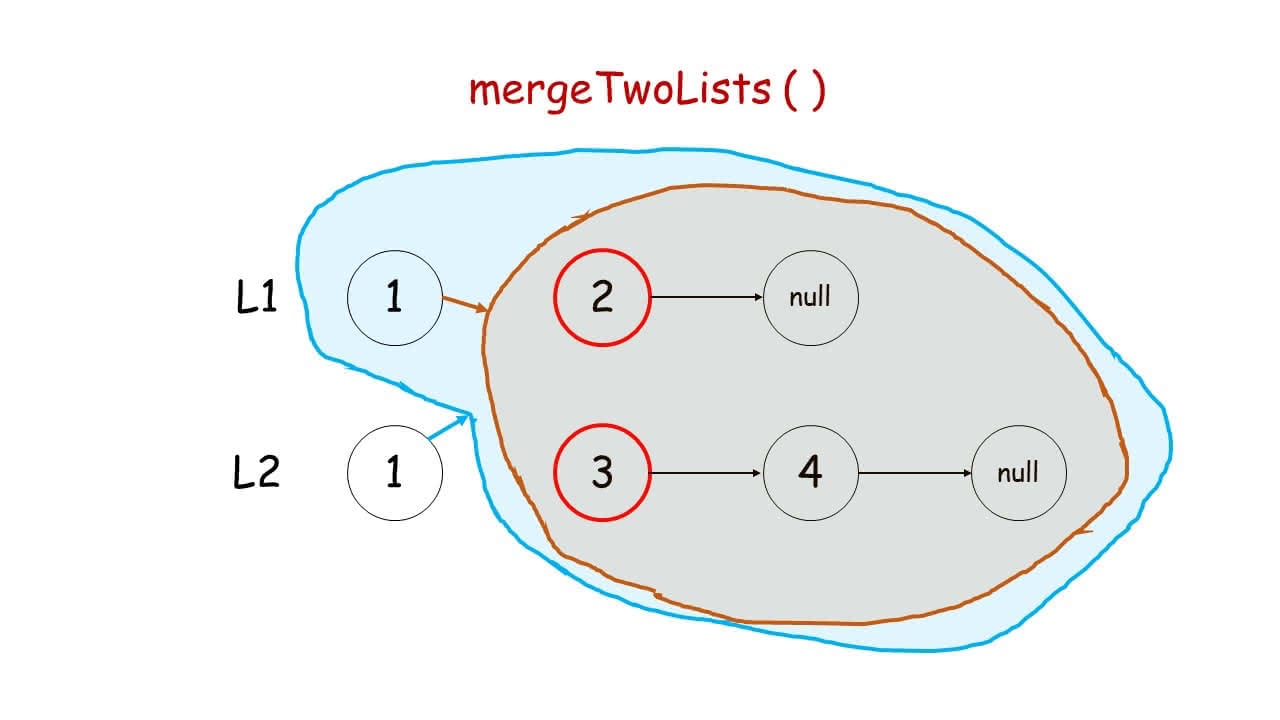
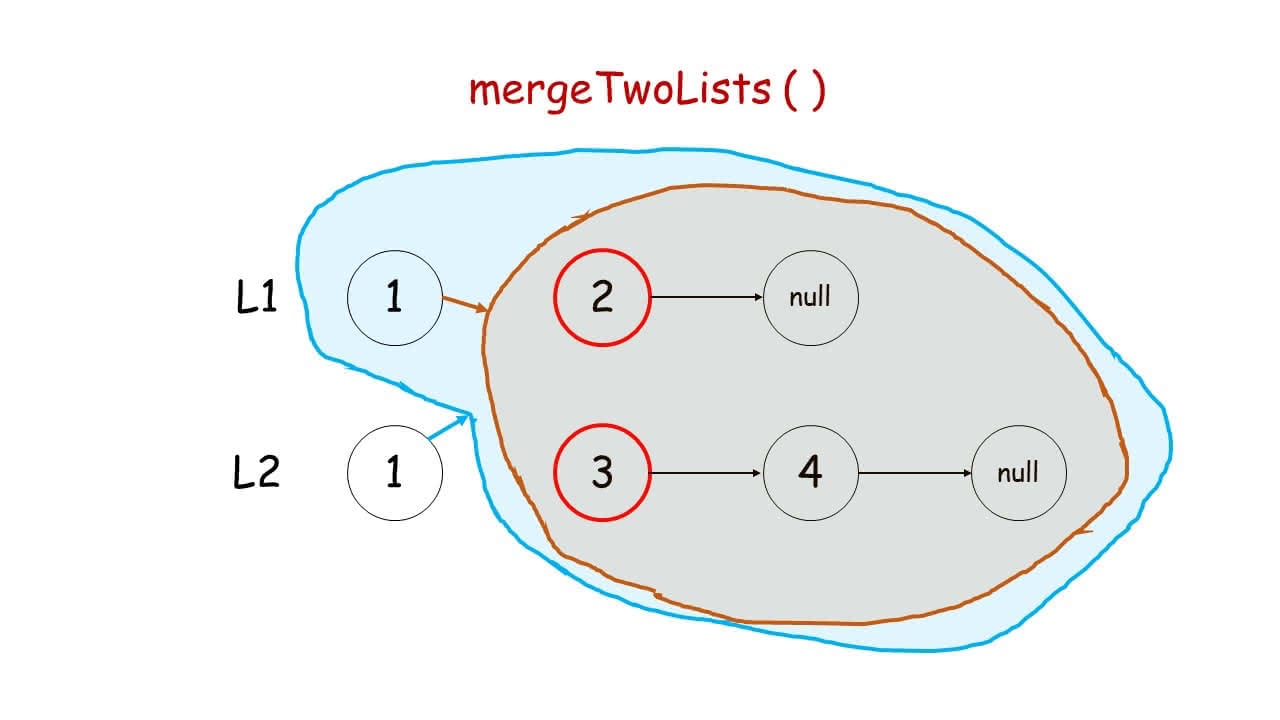
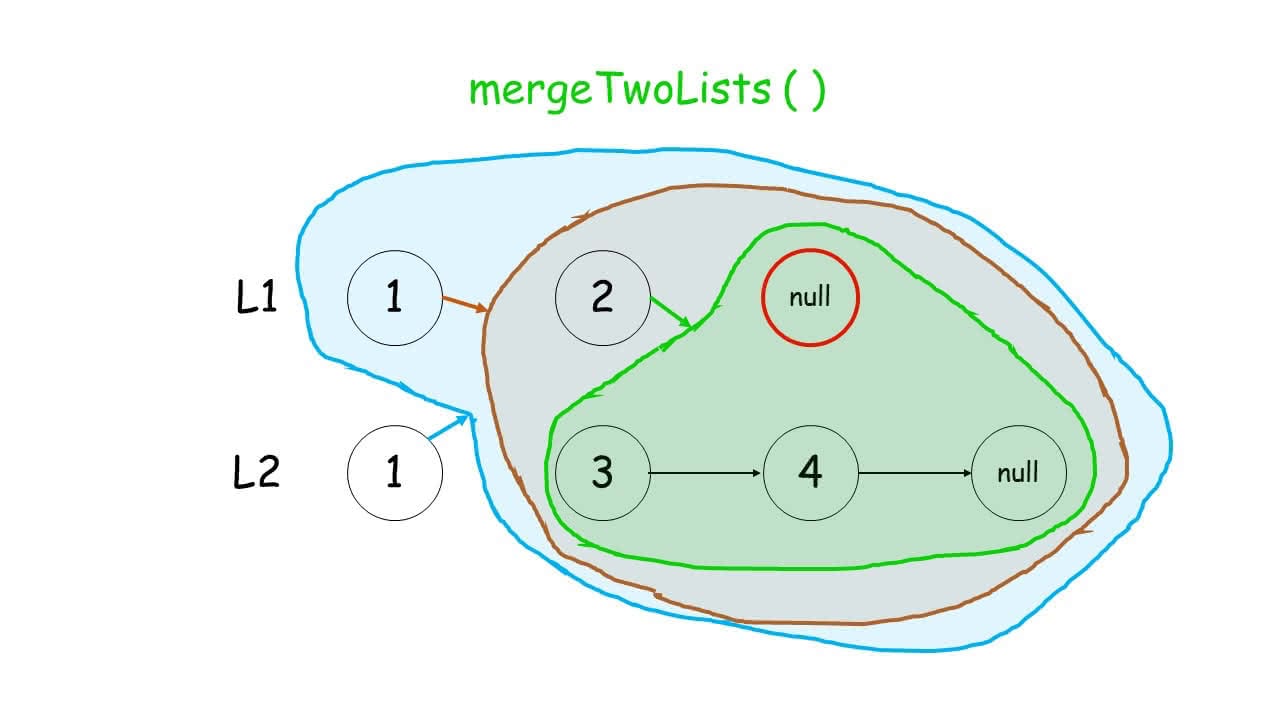
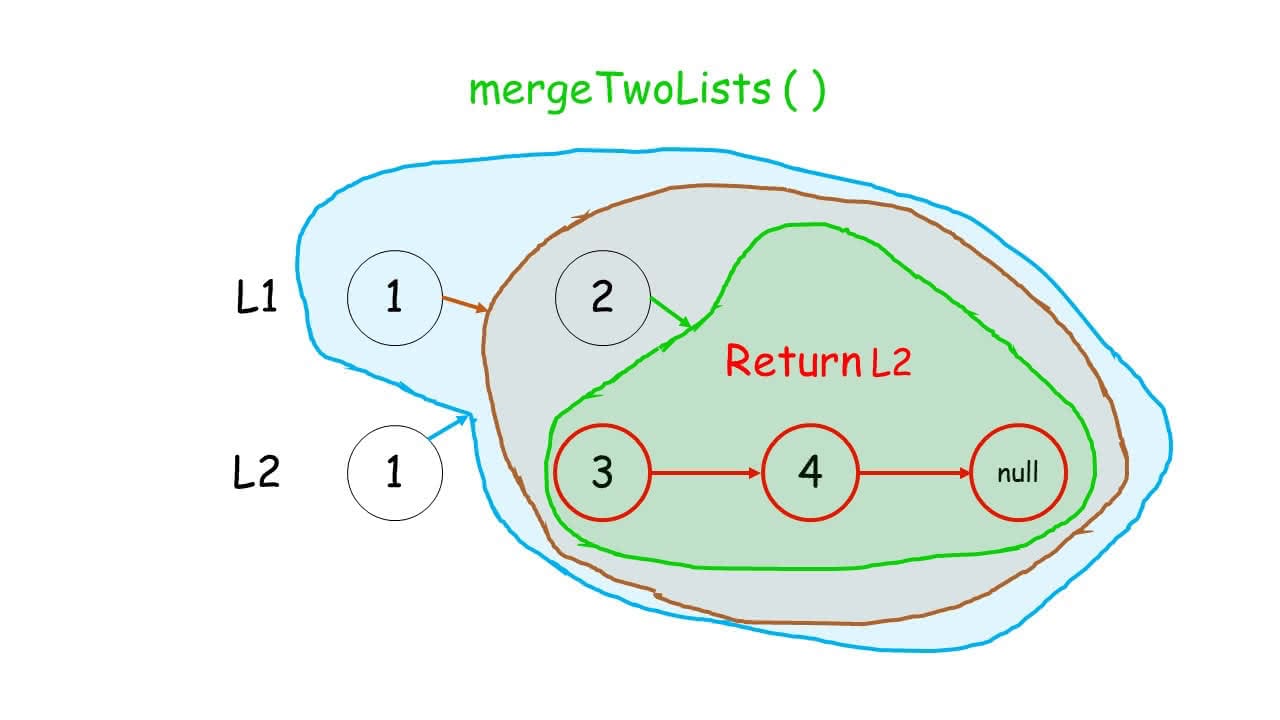
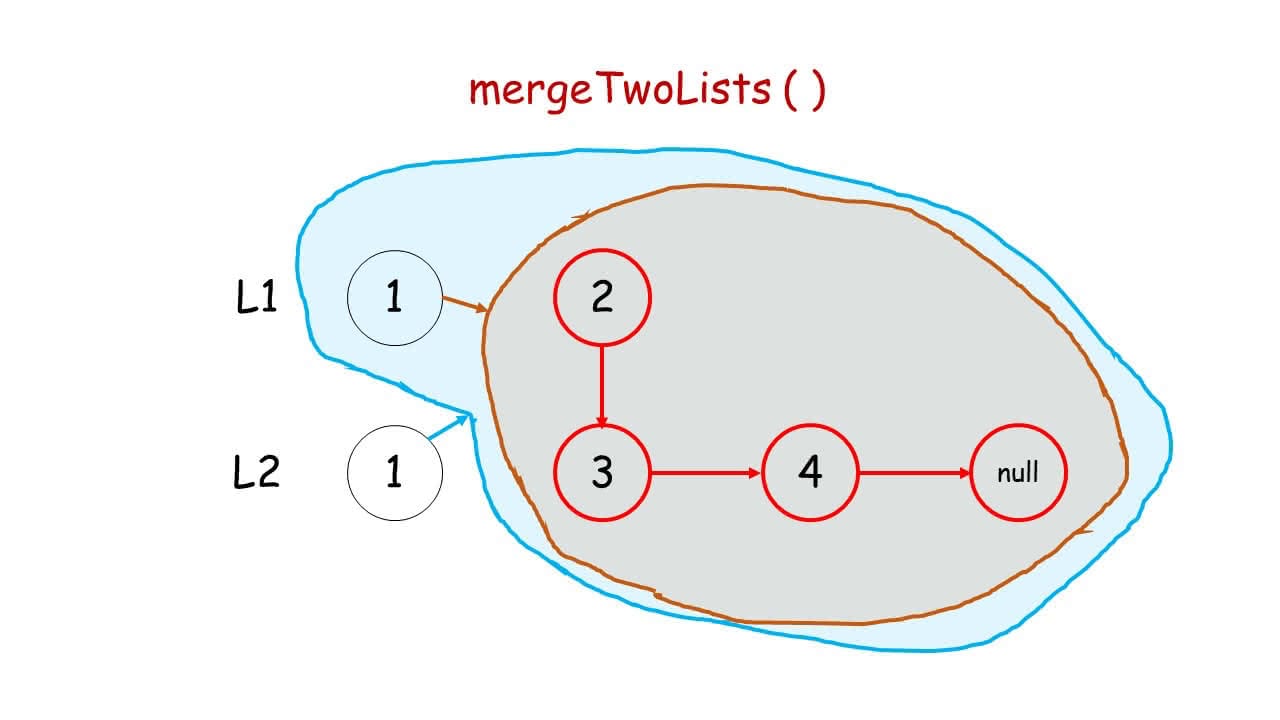
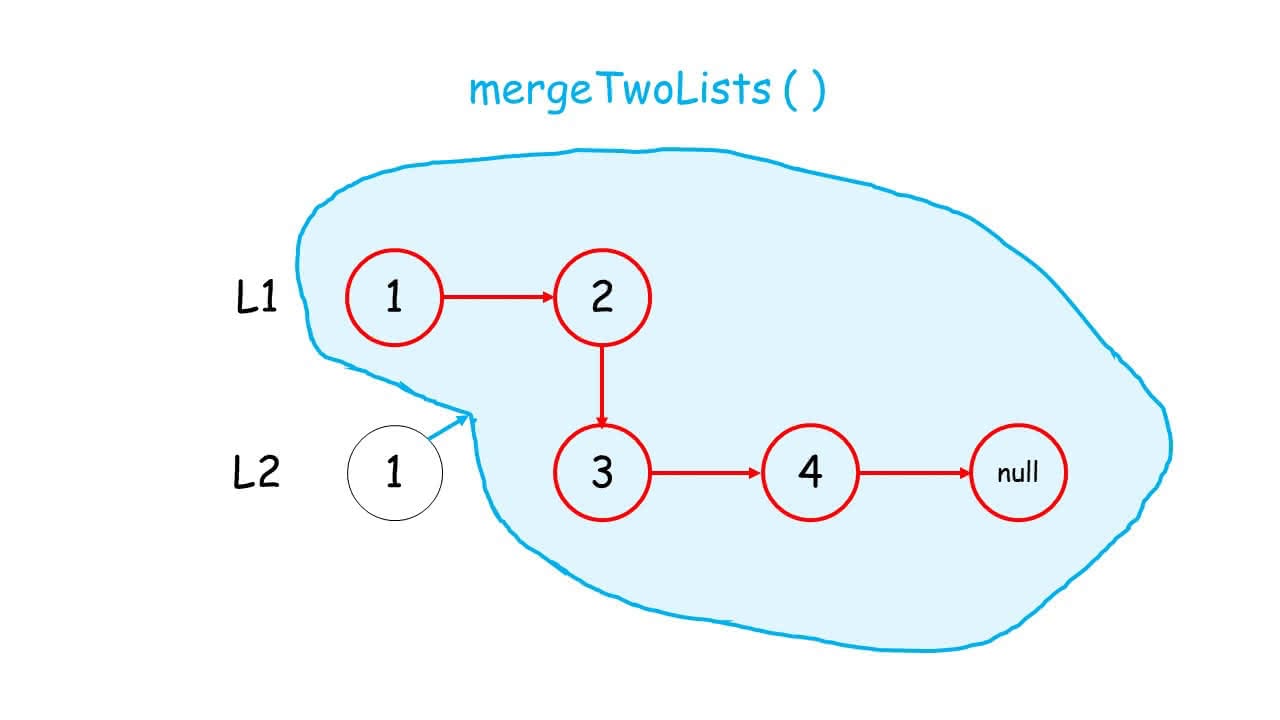
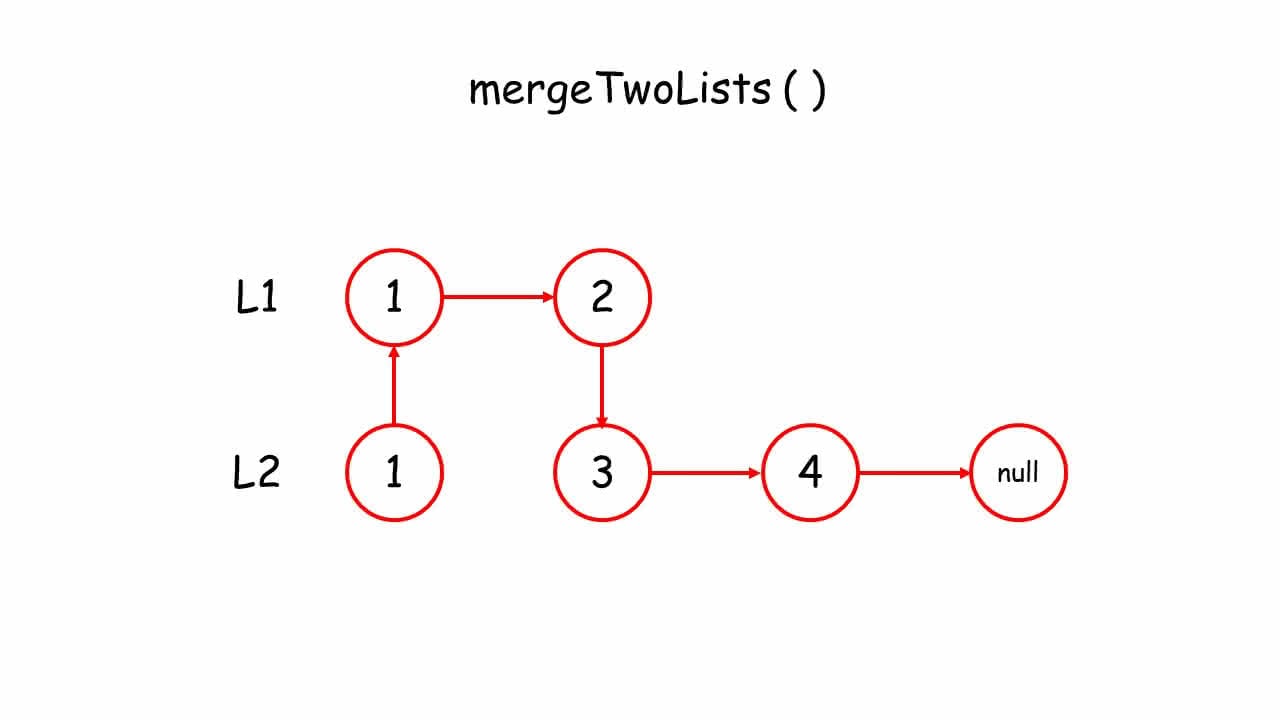
-
一刷
-
二刷
-
三刷
-
四刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
if (Objects.isNull(l1) && Objects.isNull(l2)) {
return null;
}
ListNode dummy = new ListNode();
ListNode tail = dummy;
while (Objects.nonNull(l1) && Objects.nonNull(l2)) {
if (l1.val < l2.val) {
tail.next = l1;
l1 = l1.next;
} else {
tail.next = l2;
l2 = l2.next;
}
tail = tail.next;
}
if (Objects.isNull(l1)) {
tail.next = l2;
}
if (Objects.isNull(l2)) {
tail.next = l1;
}
return dummy.next;
}
/**
* Runtime: 1 ms, faster than 28.15% of Java online submissions for Merge Two Sorted Lists.
*
* Memory Usage: 39.8 MB, less than 14.45% of Java online submissions for Merge Two Sorted Lists.
*/
public ListNode mergeTwoLists1(ListNode l1, ListNode l2) {
if (Objects.isNull(l1)) {
return l2;
}
if (Objects.isNull(l2)) {
return l1;
}
ListNode result = null;
ListNode p1 = l1;
ListNode p2 = l2;
ListNode tail = null;
while (Objects.nonNull(p1) && Objects.nonNull(p2)) {
int v1 = p1.val;
int v2 = p2.val;
ListNode temp = null;
if (v1 < v2) {
temp = p1;
p1 = p1.next;
} else {
temp = p2;
p2 = p2.next;
}
if (Objects.isNull(tail)) {
result = temp;
tail = temp;
} else {
tail.next = temp;
tail = temp;
}
}
if (Objects.isNull(p1)) {
tail.next = p2;
}
if (Objects.isNull(p2)) {
tail.next = p1;
}
return result;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-07-02 19:43:59
*/
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
if (Objects.isNull(l1)) {
return l2;
}
if (Objects.isNull(l2)) {
return l1;
}
ListNode dummy = new ListNode();
ListNode curr = dummy;
while (l1 != null && l2 != null) {
if (l1.val <= l2.val) {
curr.next = l1;
l1 = l1.next;
} else if (l2.val < l1.val) {
curr.next = l2;
l2 = l2.next;
}
curr = curr.next;
if (l1 == null) {
curr.next = l2;
break;
}
if (l2 == null) {
curr.next = l1;
break;
}
}
return dummy.next;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-07-02 19:43:59
*/
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
if (l1 == null) {
return l2;
} else if (l2 == null) {
return l1;
} else if (l1.val < l2.val) {
// 升序链表,则把最小的留下,其余的进行递归。
l1.next = mergeTwoLists(l1.next, l2);
return l1;
} else {
l2.next = mergeTwoLists(l1, l2.next);
return l2;
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-04-05 23:39:31
*/
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
ListNode dummy = new ListNode(0);
ListNode cur = dummy;
while (l1 != null && l2 != null) {
if (l1.val <= l2.val) {
cur.next = l1;
l1 = l1.next;
} else {
cur.next = l2;
l2 = l2.next;
}
cur = cur.next;
}
if (l1 != null) {
cur.next = l1;
}
if (l2 != null) {
cur.next = l2;
}
return dummy.next;
}