友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
237. Delete Node in a Linked List
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.
Given linked list — head = [4,5,1,9], which looks like following:

Example 1:
Input: head = [4,5,1,9], node = 5
Output: [4,1,9]
Explanation: You are given the second node with value 5, the linked list should become 4 -> 1 -> 9 after calling your function.
Example 2:
Input: head = [4,5,1,9], node = 1
Output: [4,5,9]
Explanation: You are given the third node with value 1, the linked list should become 4 -> 5 -> 9 after calling your function.
Note:
-
The linked list will have at least two elements.
-
All of the nodes' values will be unique.
-
The given node will not be the tail and it will always be a valid node of the linked list.
-
Do not return anything from your function.
思路分析
这个题其实很简单!把节点的值覆盖当前节点的值即可。
没想到打脸如此之快!还有更简单的办法,两行代码搞定:①把下一个节点的值拷贝到当前节点;②把当前节点的下一节点指向下下一个节点即可。
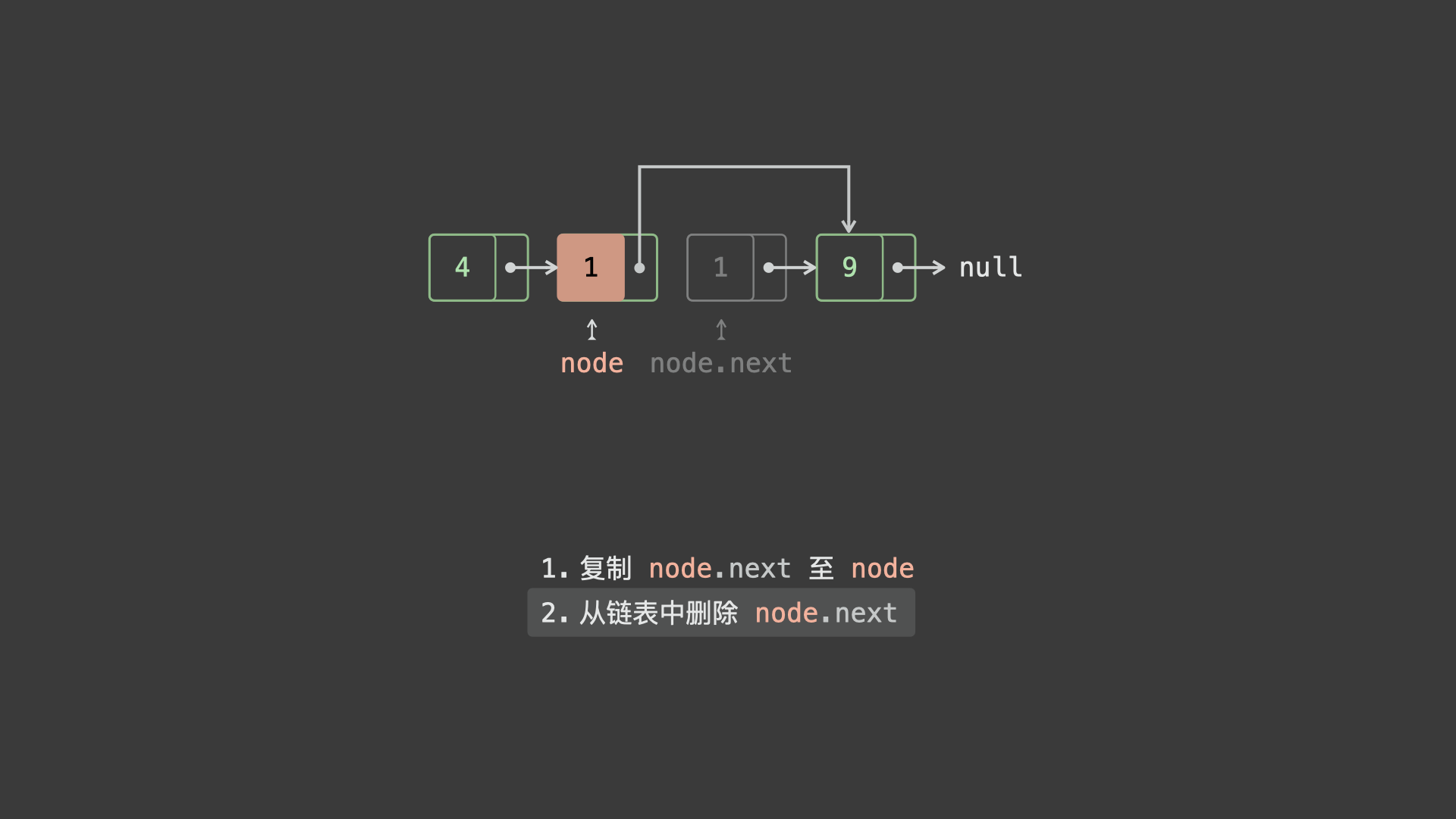
-
一刷
-
二刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Delete Node in a Linked List.
*
* Memory Usage: 36.2 MB, less than 100.00% of Java online submissions for Delete Node in a Linked List.
*
* Copy from: https://leetcode.com/problems/delete-node-in-a-linked-list/solution/[Delete Node in a Linked List solution - LeetCode]
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-01-13 20:28
*/
public void deleteNode(ListNode node) {
node.val = node.next.val;
node.next = node.next.next;
}
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Delete Node in a Linked List.
*
* Memory Usage: 36.5 MB, less than 100.00% of Java online submissions for Delete Node in a Linked List.
*/
public void deleteNodeCopyList(ListNode node) {
ListNode current = node;
while (Objects.nonNull(current) && Objects.nonNull(current.next)) {
ListNode next = current.next;
current.val = next.val;
if (Objects.nonNull(next.next)) {
current = next;
} else {
current.next = null;
}
}
}
1
2
3
4
5
6
7
8
9
10
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-16 20:54:33
*/
public void deleteNode(ListNode node) {
// 把后面一个元素拷贝过来
node.val = node.next.val;
// 把当前元素的下节点指针指向下下节点
node.next = node.next.next;
}