友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
151. 反转字符串中的单词
给你一个字符串 s
,请你反转字符串中 单词 的顺序。
单词 是由非空格字符组成的字符串。s
中使用至少一个空格将字符串中的 单词 分隔开。
返回 单词 顺序颠倒且 单词 之间用单个空格连接的结果字符串。
注意:输入字符串 `s`中可能会存在前导空格、尾随空格或者单词间的多个空格。返回的结果字符串中,单词间应当仅用单个空格分隔,且不包含任何额外的空格。
示例 1:
输入:s = "the sky is blue" 输出:"blue is sky the"
示例 2:
输入:s = " hello world " 输出:"world hello" 解释:反转后的字符串中不能存在前导空格和尾随空格。
示例 3:
输入:s = "a good example" 输出:"example good a" 解释:如果两个单词间有多余的空格,反转后的字符串需要将单词间的空格减少到仅有一个。
提示:
-
1 <= s.length <= 104
-
s
包含英文大小写字母、数字和空格' '
-
s
中 至少存在一个 单词
进阶:如果字符串在你使用的编程语言中是一种可变数据类型,请尝试使用 \(O(1)\) 额外空间复杂度的 原地 解法。
思路分析
使用空格切分字符串,然后将字符串对调,再合并。看答案,有个一个更高效的办法,从后面分割单词,这样就不需要对调了。
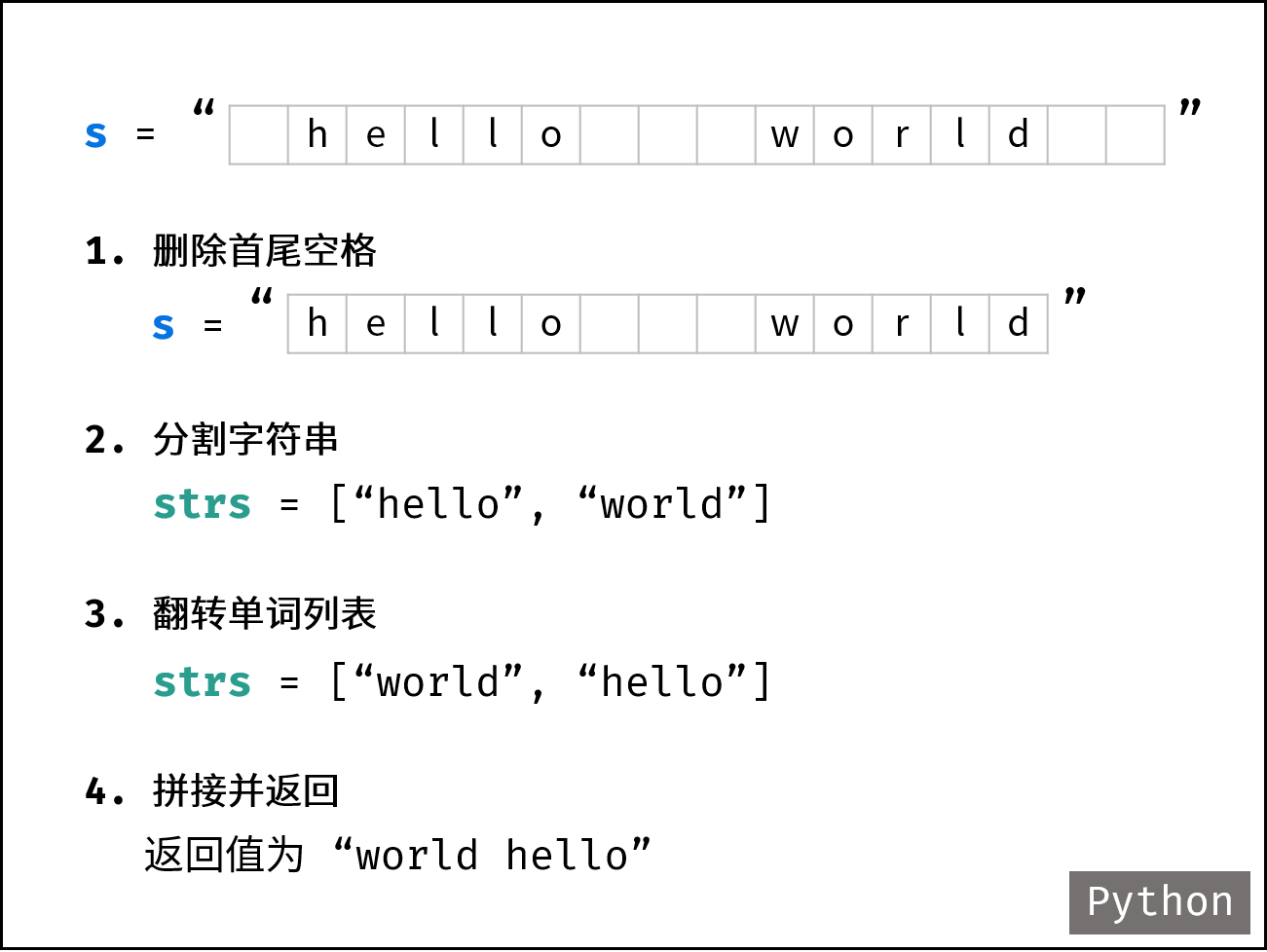
-
一刷
-
二刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-19 17:11:20
*/
public String reverseWords(String s) {
Deque<String> stack = new LinkedList<>();
boolean inWord = false;
for (char c : s.toCharArray()) {
if (c == ' ') {
inWord = false;
} else {
if (!inWord) {
stack.push(String.valueOf(c));
inWord = true;
} else {
stack.push(stack.pop() + c);
}
}
}
StringBuilder sb = new StringBuilder(s.length());
while (!stack.isEmpty()) {
sb.append(stack.pop()).append(" ");
}
sb.deleteCharAt(sb.length() - 1);
return sb.toString();
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-06-09 21:36:52
*/
public String reverseWords(String s) {
List<String> result = new ArrayList<>();
int idx = 0;
while (idx < s.length()) {
int end = s.indexOf(" ", idx);
if (end == -1) {
result.add(s.substring(idx));
break;
} else if (idx == end) {
idx++;
} else {
result.add(s.substring(idx, end));
idx = end + 1;
}
}
int left = 0, right = result.size() - 1;
while (left < right) {
String word = result.get(left);
result.set(left, result.get(right));
result.set(right, word);
left++;
right--;
}
return String.join(" ", result);
}