友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
91. 解码方法
一条包含字母 A-Z
的消息通过以下映射进行了 编码 :
"1" -> 'A'
"2" -> 'B'
...
"25" -> 'Y'
"26" -> 'Z'
然而,在 解码 已编码的消息时,你意识到有许多不同的方式来解码,因为有些编码被包含在其它编码当中(2
和 5
与 25
)。
例如,11106
可以映射为:
-
AAJF
,将消息分组为(1, 1, 10, 6)
-
KJF
,将消息分组为(11, 10, 6)
-
消息不能分组为
(1, 11, 06)
,因为06
不是一个合法编码(只有 "6" 是合法的)。
注意,可能存在无法解码的字符串。
给你一个只含数字的 非空 字符串 s
,请计算并返回 解码 方法的 总数。如果没有合法的方式解码整个字符串,返回 0
。
题目数据保证答案肯定是一个 32 位 的整数。
示例 1:
输入:s = "12" 输出:2 解释:它可以解码为 "AB"(1 2)或者 "L"(12)。
示例 2:
输入:s = "226" 输出:3 解释:它可以解码为 "BZ" (2 26), "VF" (22 6), 或者 "BBF" (2 2 6) 。
示例 3:
输入:s = "06" 输出:0 解释:"06" 无法映射到 "F" ,因为存在前导零("6" 和 "06" 并不等价)。
提示:
-
1 <= s.length <= 100
-
s
只包含数字,并且可能包含前导零。
思路分析
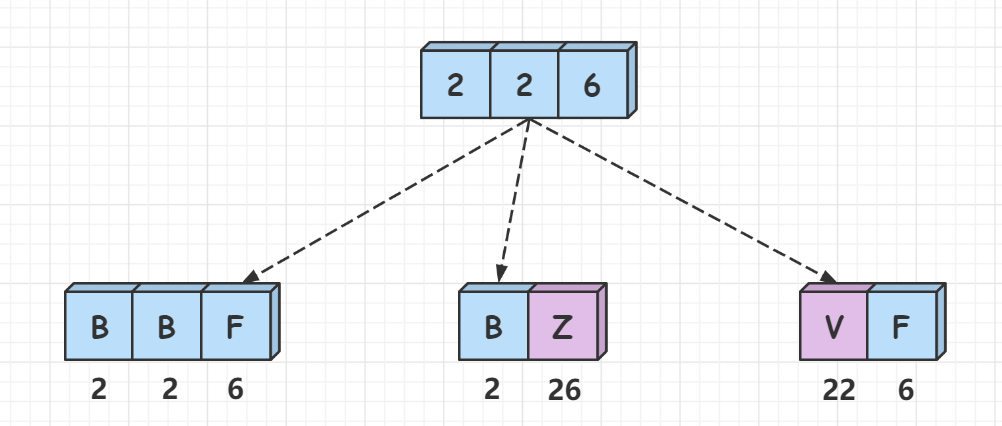
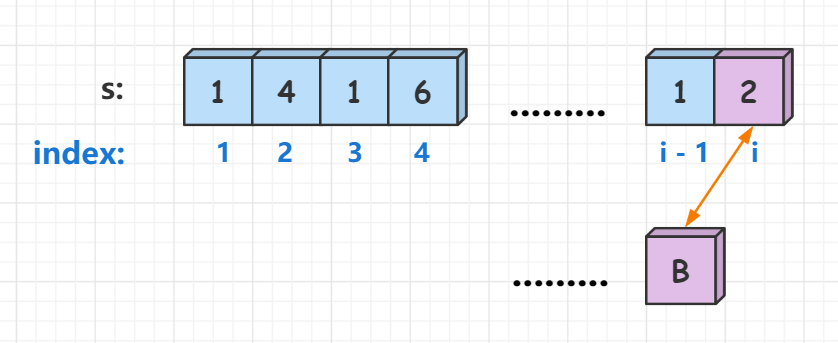
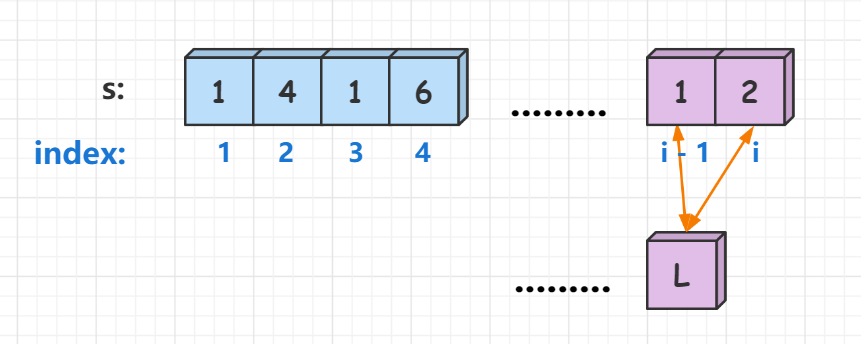
这道题可以使用动态规划算法解决。学习《算法导论》中"动态规划"的解题步骤,再推演几次这个解题方法。
-
一刷
-
二刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
/**
* Runtime: 3 ms, faster than 19.41% of Java online submissions for Decode Ways.
*
* Memory Usage: 41.5 MB, less than 5.66% of Java online submissions for Decode Ways.
*
* Copy from: https://leetcode.com/problems/decode-ways/discuss/30357/DP-Solution-(Java)-for-reference[DP Solution (Java) for reference - LeetCode Discuss]
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-01-18 00:20
*/
public int numDecodings(String s) {
if (Objects.isNull(s) || s.isEmpty()) {
return 0;
}
int length = s.length();
int[] memo = new int[length + 1];
memo[length] = 1;
memo[length - 1] = s.charAt(length - 1) != '0' ? 1 : 0;
for (int i = length - 2; i >= 0; i--) {
if (s.charAt(i) == '0') {
continue;
} else {
int value = Integer.parseInt(s.substring(i, i + 2));
memo[i] = value <= 26 ? memo[i + 1] + memo[i + 2] : memo[i + 1];
}
}
return memo[0];
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-05-20 16:13:52
*/
public int numDecodings(String s) {
if (s.charAt(0) == '0') {
return 0;
}
int[] dp = new int[s.length() + 1];
dp[0] = 1; // 没有字符也是一种解码方案(主要是为了保证后续递推的正确性)
for (int i = 1; i <= s.length(); i++) {
int val = s.charAt(i - 1) - '0';
if (val != 0) {
dp[i] = dp[i - 1];
}
if (i >= 2) {
int pre = (s.charAt(i - 2) - '0') * 10;
int sum = pre + val;
if (sum >= 10 && sum <= 26) {
dp[i] += dp[i - 2];
}
}
}
return dp[s.length()];
}