友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
724. Find Pivot Index
Given an array of integers nums
, write a method that returns the "pivot" index of this array.
We define the pivot index as the index where the sum of the numbers to the left of the index is equal to the sum of the numbers to the right of the index.
If no such index exists, we should return -1. If there are multiple pivot indexes, you should return the left-most pivot index.
Example 1:
Input:
nums = [1, 7, 3, 6, 5, 6]
Output: 3
Explanation:
The sum of the numbers to the left of index 3 (nums[3] = 6) is equal to the sum of numbers to the right of index 3.
Also, 3 is the first index where this occurs.
Example 2:
Input:
nums = [1, 2, 3]
Output: -1
Explanation:
There is no index that satisfies the conditions in the problem statement.
Note:
-
The length of
nums
will be in the range[0, 10000]
. -
Each element
nums[i]
will be an integer in the range[-1000, 1000]
.
思路分析
这道题的技巧和 238. Product of Array Except Self 类似。
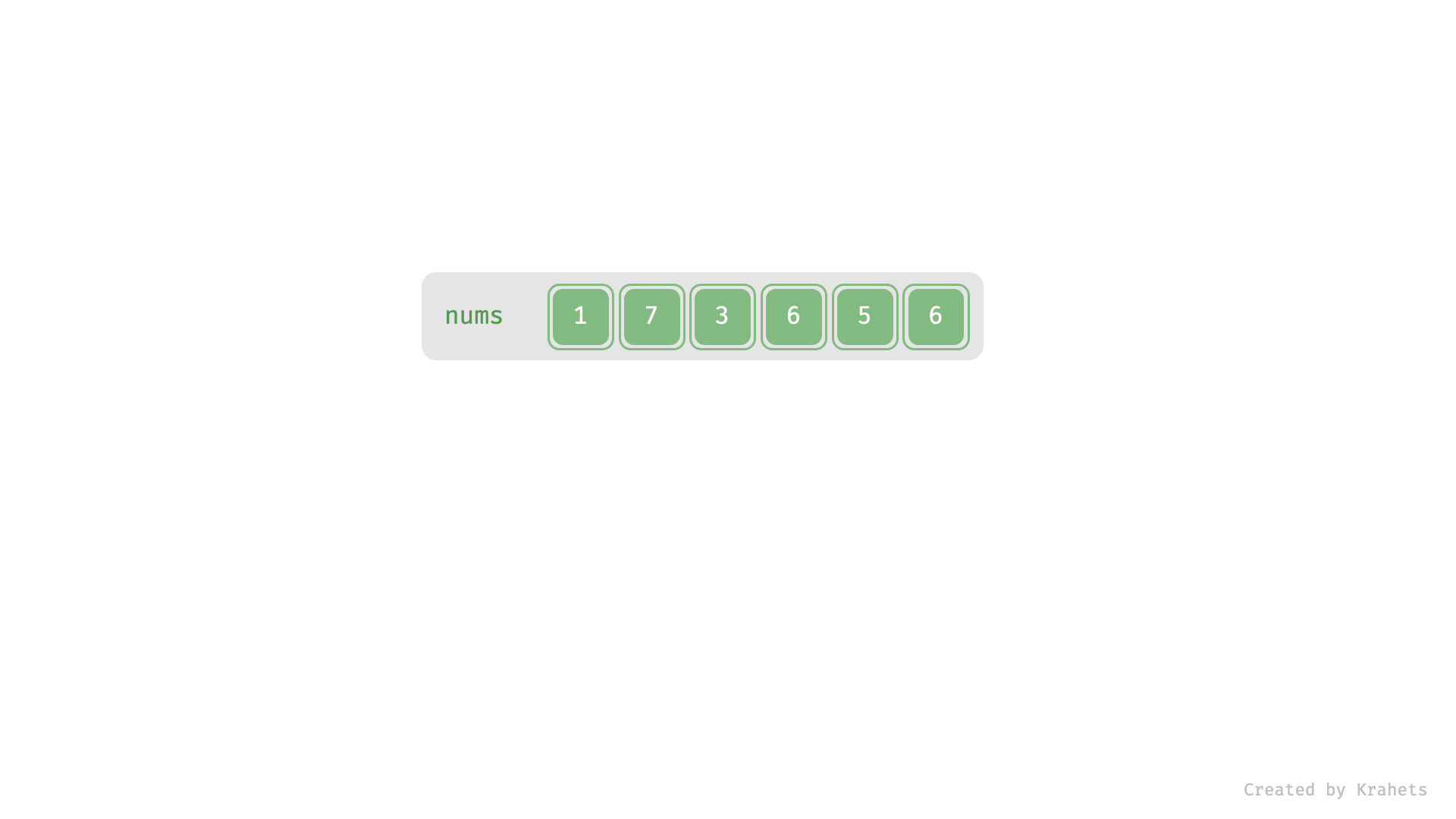
看答案,也可以直接求和,然后在依次相加减得到两边的和。
-
一刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-18 21:45:49
*/
public int pivotIndex(int[] nums) {
int length = nums.length;
int[] dp = new int[length + 2];
for (int i = length; i > 0; i--) {
dp[i] = nums[i - 1] + dp[i + 1];
}
for (int i = 1; i <= length; i++) {
if (dp[i - 1] == dp[i + 1]) {
return i - 1;
}
dp[i] = nums[i - 1] + dp[i - 1];
}
return -1;
}