友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
33. 搜索旋转排序数组
整数数组 nums
按升序排列,数组中的值 互不相同 。
在传递给函数之前,nums
在预先未知的某个下标 k
(0 <= k < nums.length
)上进行了 旋转,使数组变为 [nums[k], nums[k+1], ..., nums[n-1], nums[0], nums[1], ..., nums[k-1]]
(下标 从 0 开始 计数)。例如, [0,1,2,4,5,6,7]
在下标 3
处经旋转后可能变为 [4,5,6,7,0,1,2]
。
给你 旋转后 的数组 nums
和一个整数 target
,如果 nums
中存在这个目标值 target
,则返回它的下标,否则返回 -1
。
你必须设计一个时间复杂度为 \(log_2N\) 的算法解决此问题。
示例 1:
输入:nums = [4,5,6,7,0,1,2], target = 0 输出:4
示例 2:
输入:nums = [4,5,6,7,0,1,2], target = 3 输出:-1
示例 3:
输入:nums = [1], target = 0 输出:-1
提示:
-
1 <= nums.length <= 5000
-
-10+
4
<= nums[i] <= 10
4
-
nums
中的每个值都 独一无二 -
题目数据保证
nums
在预先未知的某个下标上进行了旋转 -
-10
4
<= target <= 10
4
思路分析
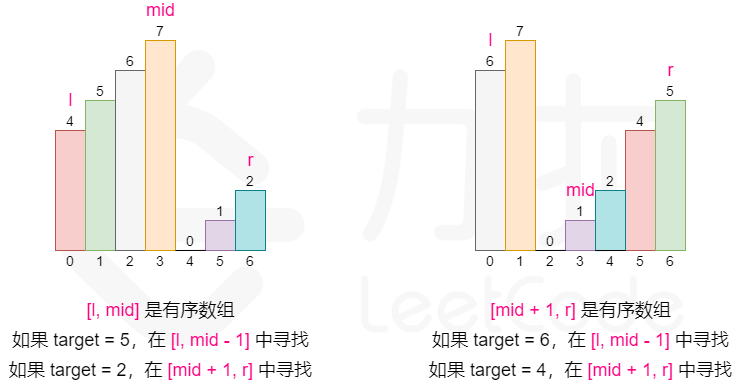
-
一刷
-
二刷
-
三刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2018-09-16 17:45
*/
public static int search(int[] nums, int target) {
int result = -1;
if (null == nums || nums.length == 0) {
return result;
}
int firstNum = nums[0];
int lastNum = nums[nums.length - 1];
int separator = -1;
if (firstNum > lastNum) {
int head = 0;
int tail = nums.length - 1;
while (head <= tail) {
int mid = head + (tail - head) / 2;
int midNum = nums[mid];
if (midNum > nums[mid + 1]) {
separator = mid;
break;
}
if (midNum >= firstNum) {
head = mid + 1;
}
if (midNum < lastNum) {
tail = mid - 1;
}
}
}
if (separator == -1) {
return binarySearch(nums, target, 0, nums.length - 1);
} else {
if (firstNum <= target && target <= nums[separator]) {
return binarySearch(nums, target, 0, separator);
} else {
return binarySearch(nums, target, separator + 1, nums.length - 1);
}
}
}
private static int binarySearch(int[] nums, int target, int headIndex, int tailIndex) {
int head = headIndex;
int tail = tailIndex;
while (head <= tail) {
int mid = head + (tail - head) / 2;
int midNum = nums[mid];
if (midNum == target) {
return mid;
}
if (target <= midNum) {
tail = mid - 1;
}
if (midNum < target) {
head = mid + 1;
}
}
return -1;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-03-05 14:11:58
*/
public int search(int[] nums, int target) {
int left = 0, right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) {
return mid;
}
if (nums[0] <= nums[mid]) {
// 由于 nums[0] <= nums[mid],所以,这个分支处理的是前面有序的情况
// --------------------------------------------
// 上面已经判断过 nums[mid] 和 target 是否相等,
// 这里就不需要再处理相等情况,所以,可以直接去 mid 左右的索引
// 该分支前面有序,只需要在有序数组里去查找即可,不满足要求,则在另外一部分里。
if (nums[0] <= target && target < nums[mid]) {
right = mid - 1;
} else {
left = mid + 1;
}
} else {
// 上面只处理前面有序的情况,那么这里就可能是后面有序的情况。
// --------------------------------------------
// 上面已经判断过 nums[mid] 和 target 是否相等,
// 这里就不需要再处理相等情况,所以,可以直接去 mid 左右的索引
// 同理,这里也只在有序的数组里去查找,不满足要求则去另外一部分查找。
if (nums[mid] < target && target <= nums[nums.length - 1]) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
return -1;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-04-06 14:59:46
*/
public int search(int[] nums, int target) {
int left = 0, right = nums.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (nums[mid] == target) {
return mid;
}
if (nums[0] <= nums[mid]) {
if (nums[left] <= target && target < nums[mid]) {
right = mid - 1;
} else {
left = mid + 1;
}
} else {
if (nums[mid] < target && target <= nums[right]) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
return -1;
}