友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
3. 无重复字符的最长子串
给定一个字符串 s
,请你找出其中不含有重复字符的 最长子串 的长度。
示例 1:
输入: s = "abcabcbb" 输出: 3 解释: 因为无重复字符的最长子串是 "abc",所以其长度为 3。
示例 2:
输入: s = "bbbbb" 输出: 1 解释: 因为无重复字符的最长子串是 "b",所以其长度为 1。
示例 3:
输入: s = "pwwkew" 输出: 3 解释: 因为无重复字符的最长子串是 "wke",所以其长度为 3。 请注意,你的答案必须是 子串 的长度,"pwke" 是一个子序列,不是子串。
提示:
-
0 <= s.length <= 5 * 104
-
s
由英文字母、数字、符号和空格组成
思路分析
滑动窗口
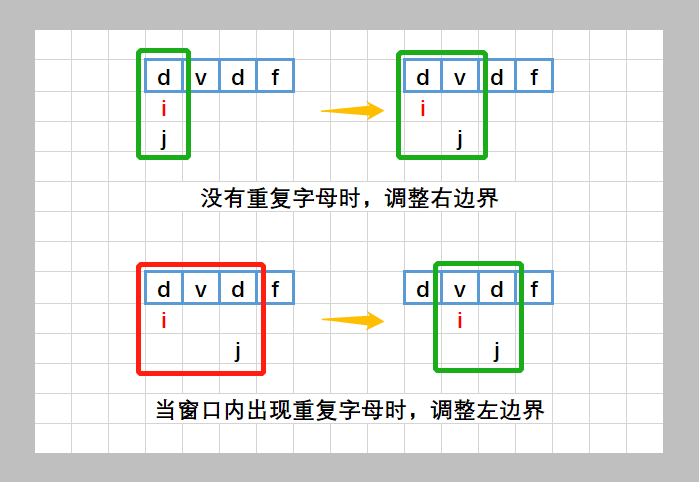
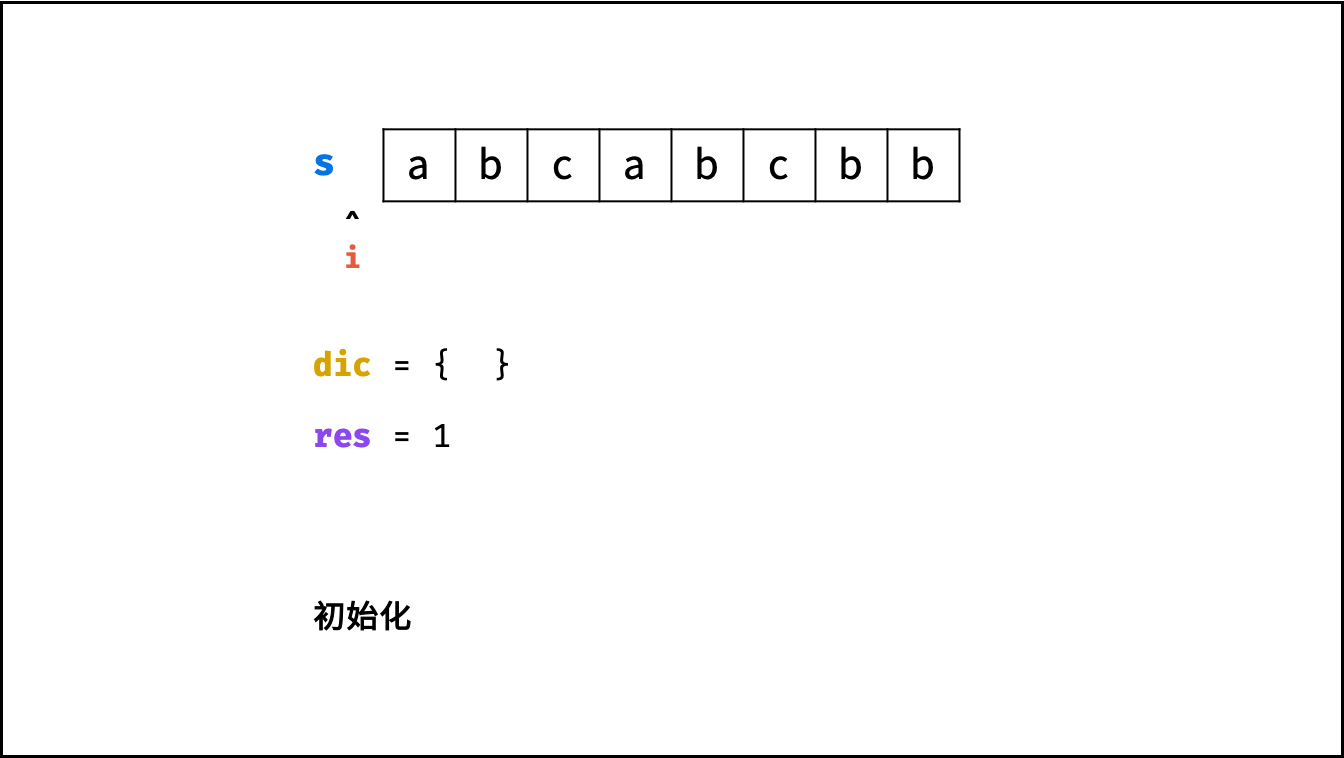
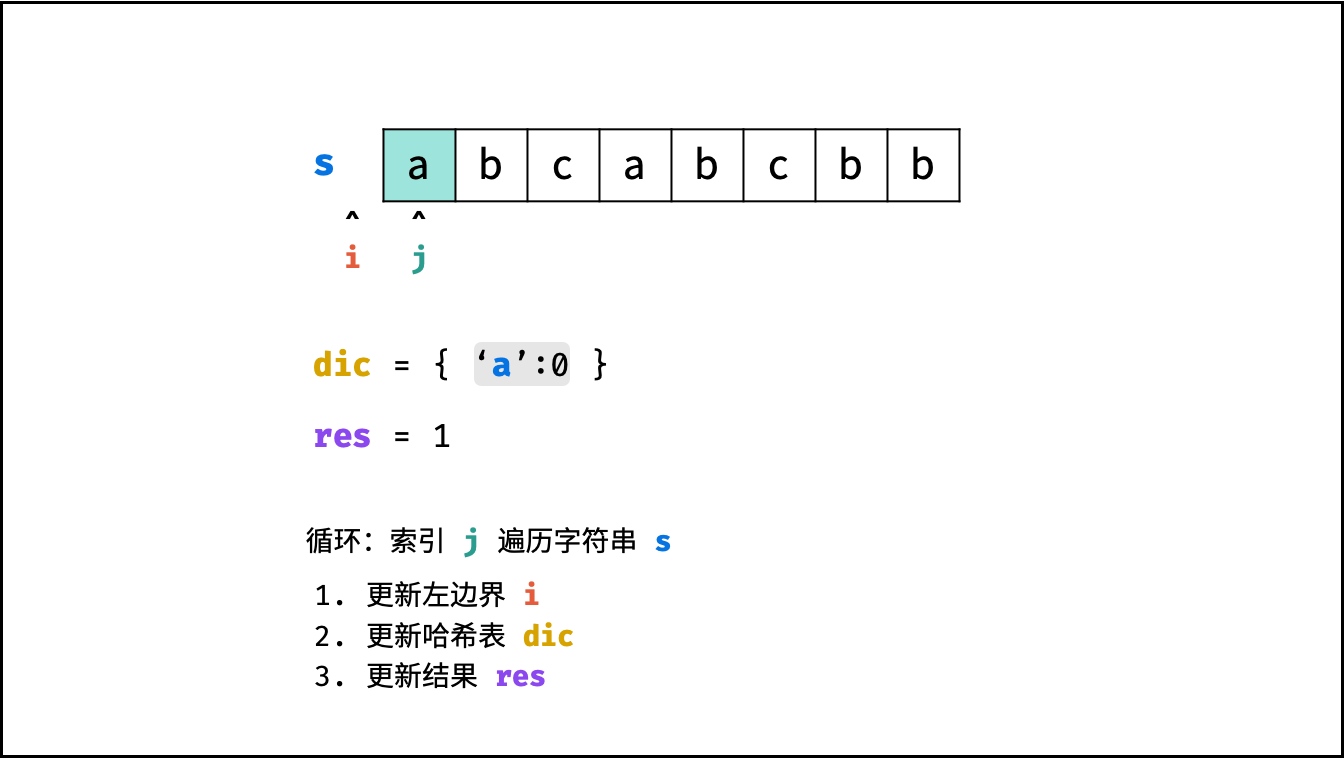
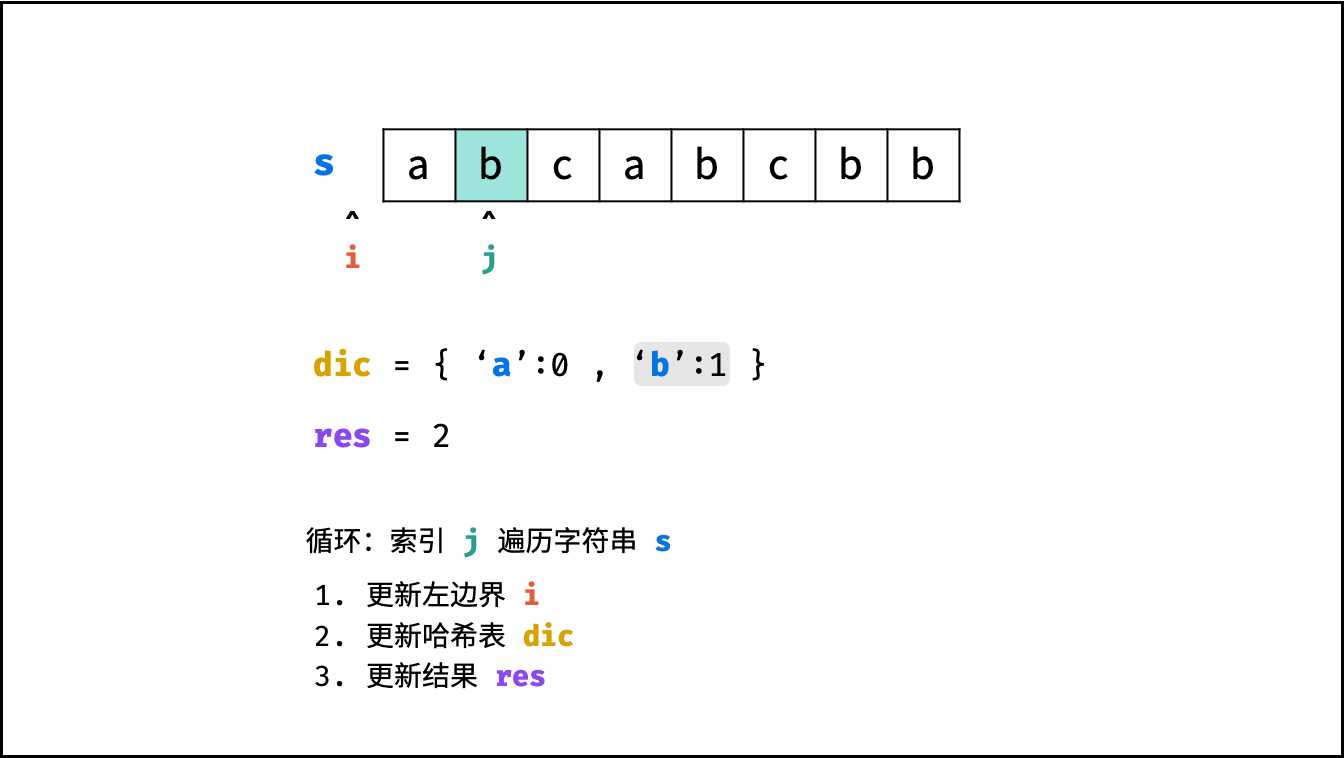
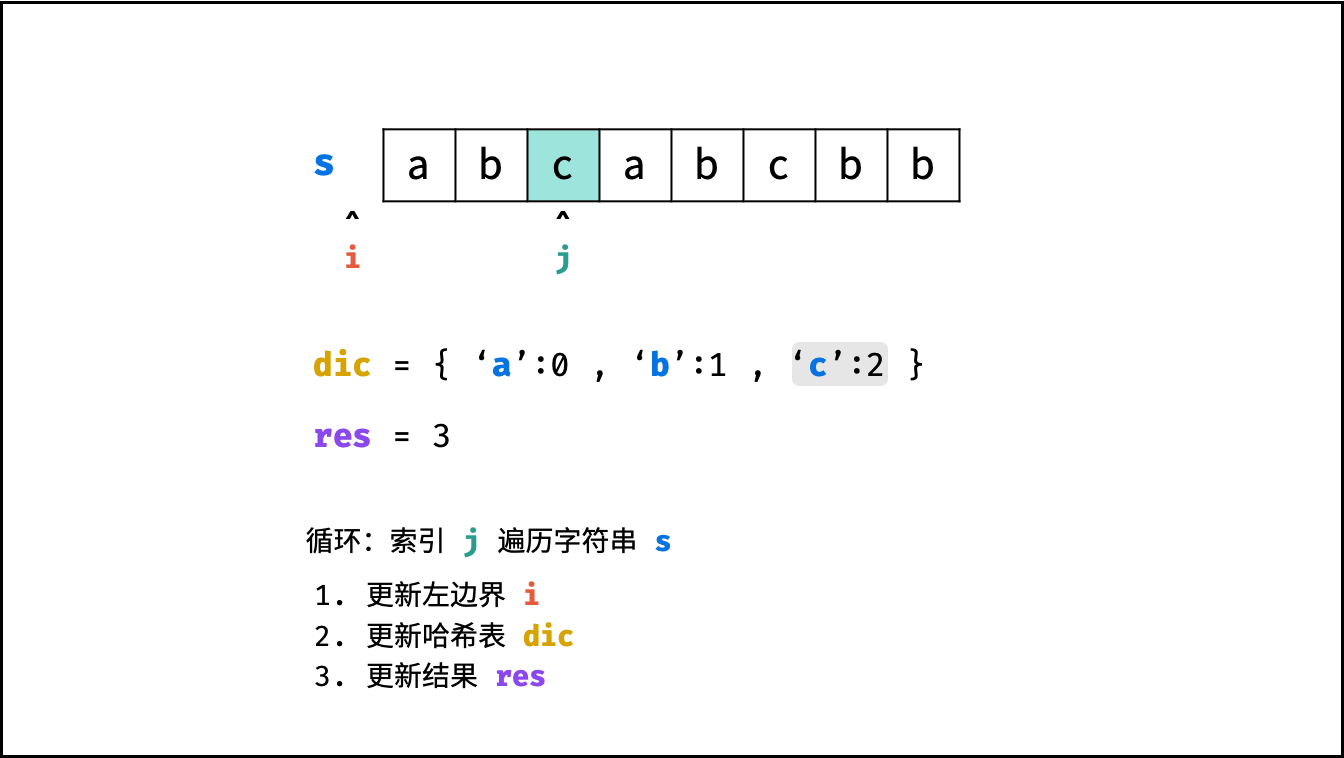
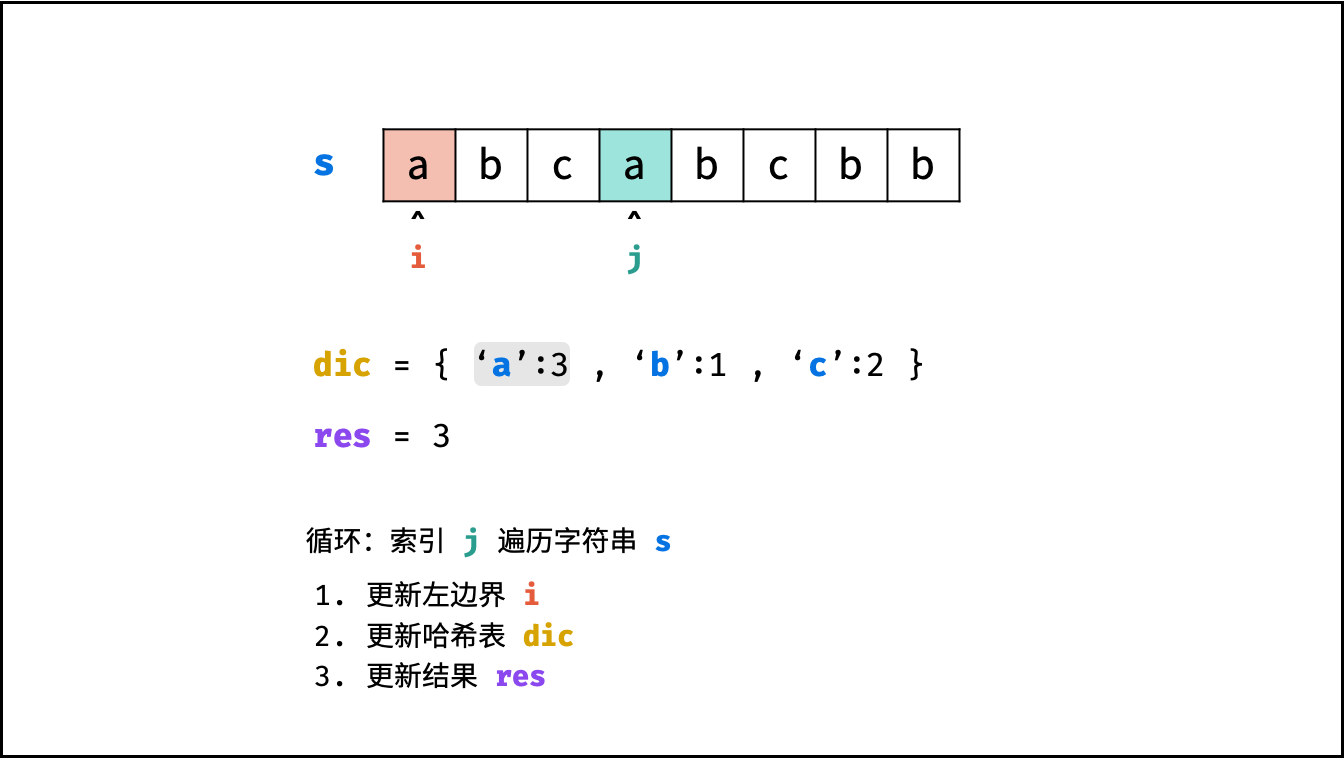
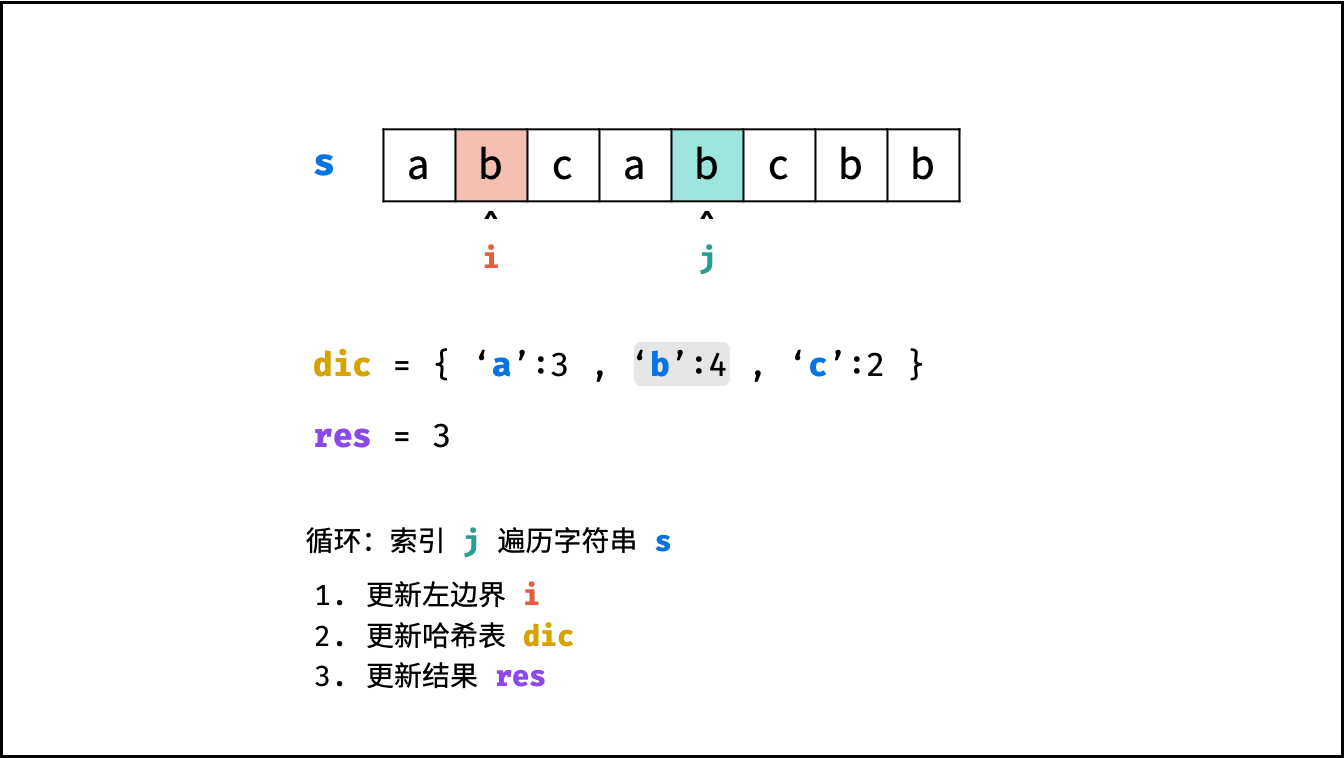
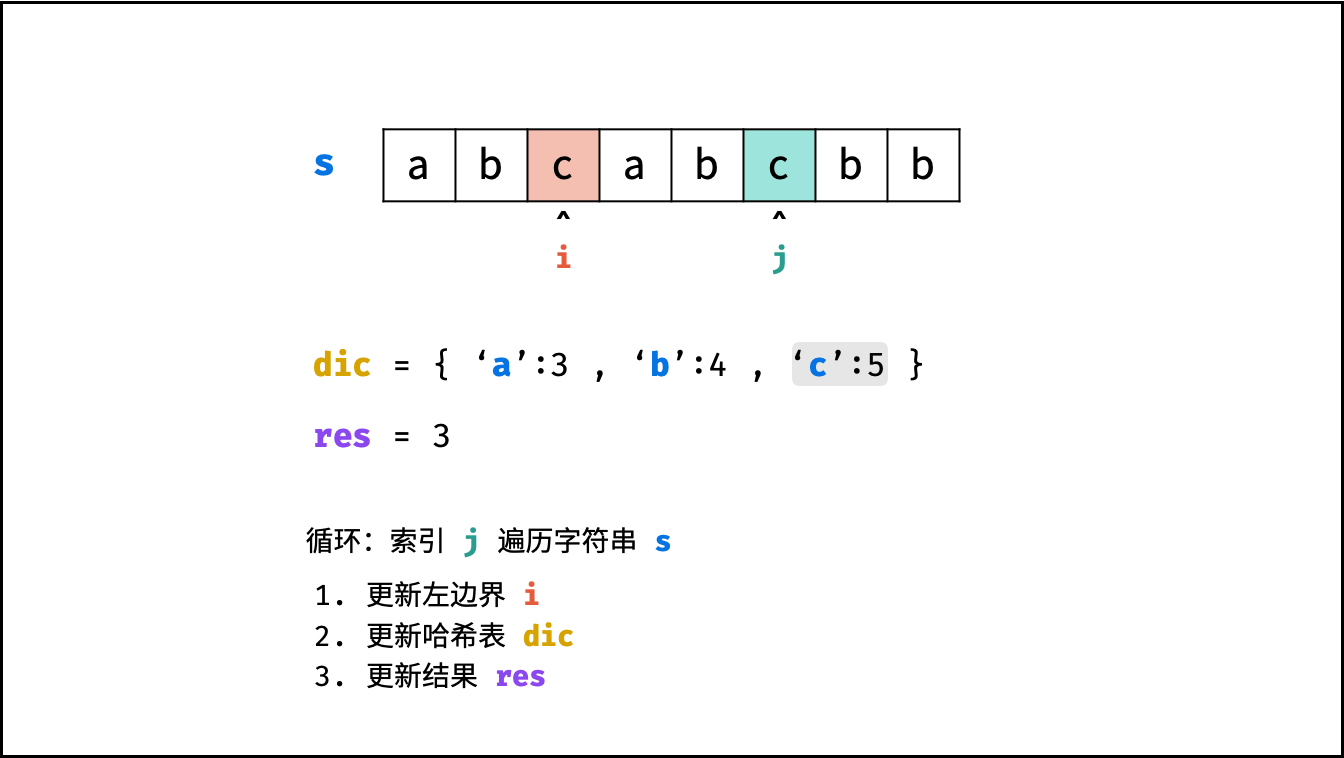
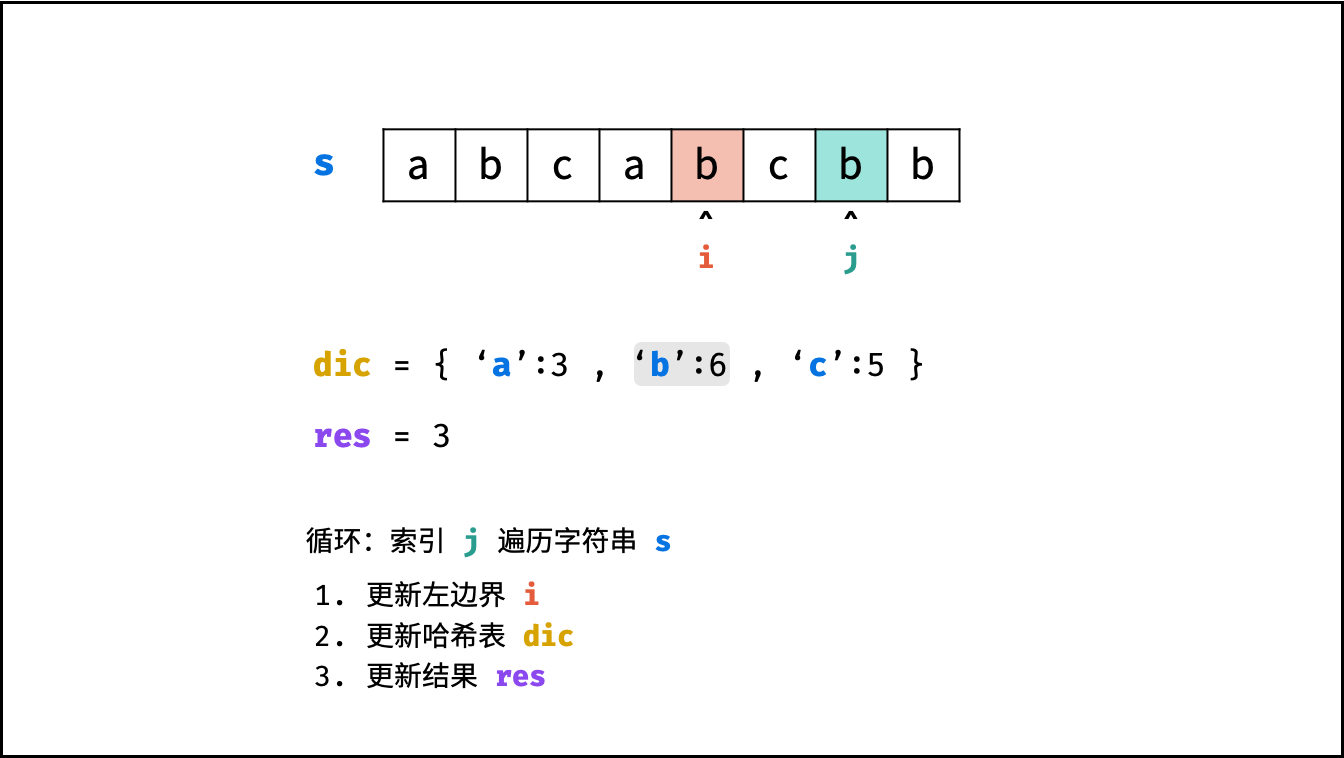
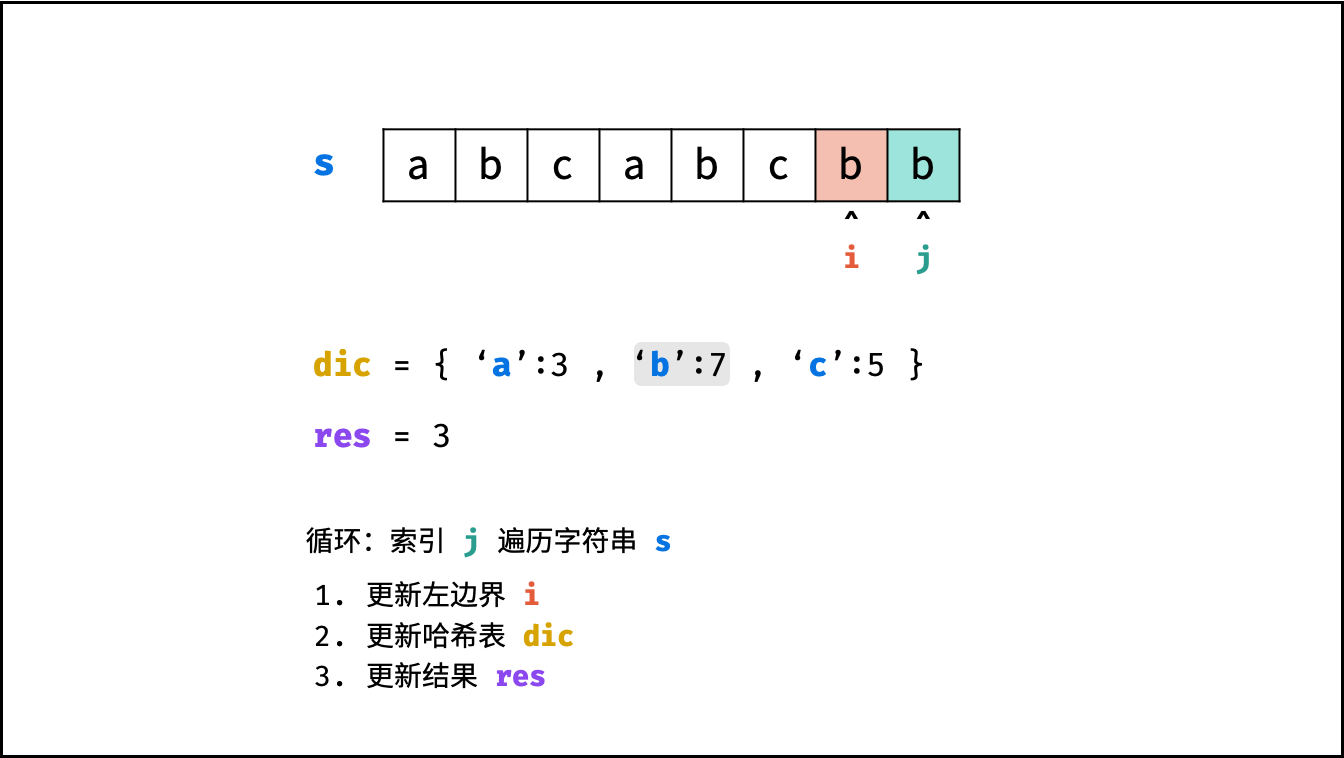
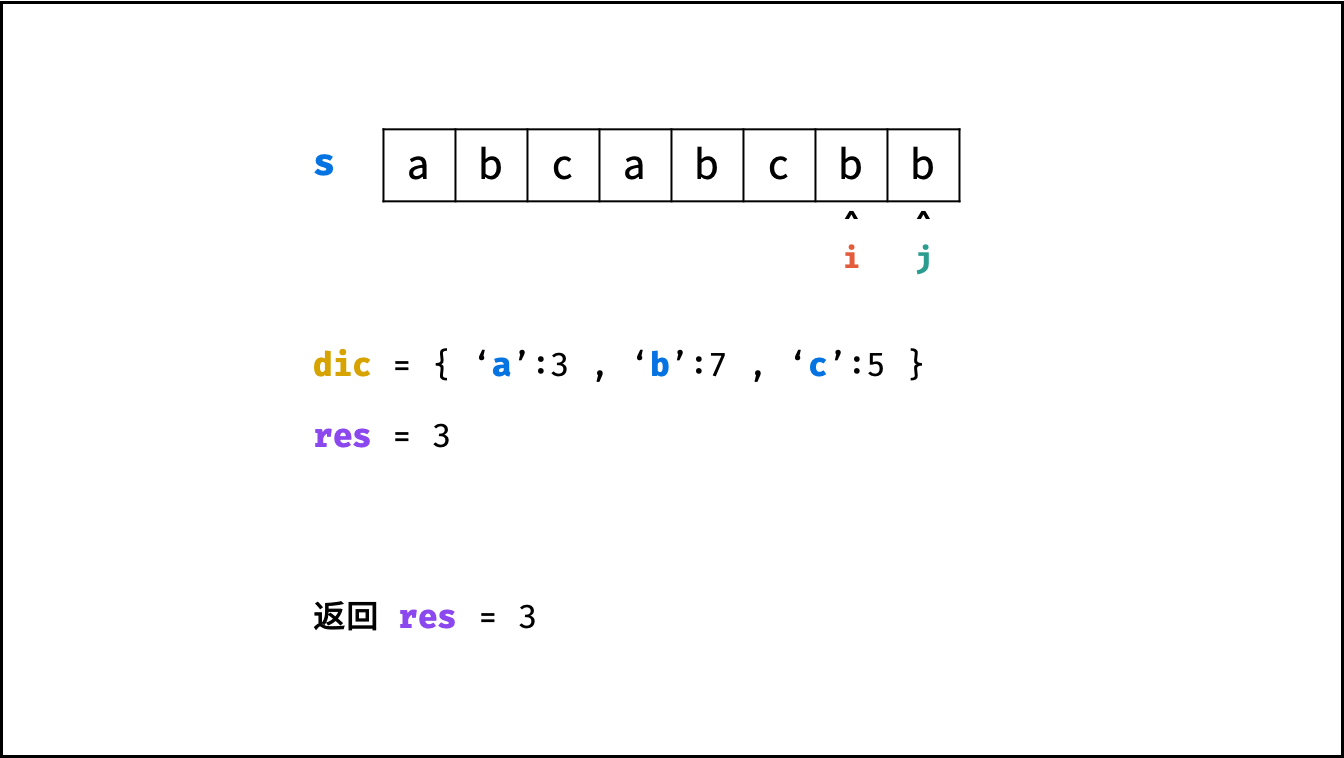
-
一刷
-
二刷
-
三刷
-
四刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
/**
* Runtime: 6 ms, faster than 85.45% of Java online submissions for Longest Substring Without Repeating Characters.
*
* Memory Usage: 36.4 MB, less than 99.80% of Java online submissions for Longest Substring Without Repeating Characters.
*
* @author D瓜哥 · https://www.diguage.com
* @since 2019-07-11 21:31
*/
public int lengthOfLongestSubstring(String s) {
if (Objects.isNull(s) || s.length() == 0) {
return 0;
}
int result = 0;
int length = s.length();
Map<Character, Integer> charToIndexMap = new HashMap<>(length * 4 / 3);
for (int i = 0, j = 0; j < length; j++) {
char aChar = s.charAt(j);
if (charToIndexMap.containsKey(aChar)) {
// 这里,如果存在重复的,则从上一个重复字符的下一个字符开始计算。
Integer index = charToIndexMap.get(aChar);
if (index + 1 > i) {
i = index + 1;
}
}
charToIndexMap.put(aChar, j);
if (j - i + 1 > result) {
result = j - i + 1;
}
}
return result;
}
/**
* Runtime: 9 ms, faster than 49.55% of Java online submissions for Longest Substring Without Repeating Characters.
*
* Memory Usage: 36 MB, less than 99.88% of Java online submissions for Longest Substring Without Repeating Characters.
*/
public int lengthOfLongestSubstringWithSlidingWindow(String s) {
if (Objects.isNull(s) || s.length() == 0) {
return 0;
}
int result = 0;
int length = s.length();
int head = 0;
int tail = 0;
Set<Character> characters = new HashSet<>(length * 4 / 3);
while (head < length && tail < length) {
if (!characters.contains(s.charAt(tail))) {
characters.add(s.charAt(tail));
tail++;
if (result < tail - head) {
result = tail - head;
}
} else {
characters.remove(s.charAt(head));
head++;
}
}
return result;
}
/**
* Runtime: 221 ms, faster than 5.02% of Java online submissions for Longest Substring Without Repeating Characters.
*
* Memory Usage: 37.3 MB, less than 97.43% of Java online submissions for Longest Substring Without Repeating Characters.
*/
public int lengthOfLongestSubstringWithBruteForce(String s) {
if (Objects.isNull(s) || s.length() == 0) {
return 0;
}
char[] chars = s.toCharArray();
int result = 0;
for (int i = 0; i < chars.length; i++) {
Set<Character> characters = new HashSet<>(chars.length * 4 / 3);
for (int j = i; j < chars.length; j++) {
char aChar = chars[j];
if (characters.contains(aChar)) {
break;
} else {
characters.add(aChar);
}
}
if (characters.size() > result) {
result = characters.size();
}
}
return result;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
/**
* 有思路,写不出代码
*
* @author D瓜哥 · https://www.diguage.com
* @since 2024-07-02 19:27:43
*/
public int lengthOfLongestSubstring(String s) {
if (s == null || s.isEmpty()) {
return 0;
}
int result = Integer.MIN_VALUE;
Map<Character, Integer> window = new HashMap<>();
int left = 0, right = 0;
while (right < s.length()) {
char rc = s.charAt(right);
right++;
window.put(rc, window.getOrDefault(rc, 0) + 1);
while (window.get(rc) > 1) {
char lc = s.charAt(left);
left++;
window.put(lc, window.getOrDefault(lc, 0) - 1);
}
result = Math.max(result, right - left);
}
return result == Integer.MAX_VALUE ? 0 : result;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-21 20:41:51
*/
public int lengthOfLongestSubstring(String s) {
Map<Character, Integer> map = new HashMap<>();
int result = 0, left = 0;
for (int right = 0; right < s.length(); right++) {
char c = s.charAt(right);
int cnt = map.getOrDefault(c, 0);
if (cnt == 0) {
map.put(c, 1);
result = Math.max(result, right - left + 1);
} else {
map.put(c, cnt + 1);
}
while (map.get(c) > 1) {
char lc = s.charAt(left);
left++;
map.put(lc, map.get(lc) - 1);
}
}
return result;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2025-04-01 17:03:21
*/
public int lengthOfLongestSubstring(String s) {
if (s == null || s.isEmpty()) {
return 0;
}
int result = 0;
Map<Character, Integer> counter = new HashMap<>();
int left = 0, right = 0;
char[] chars = s.toCharArray();
while (right < chars.length) {
char rc = chars[right];
right++;
int cnt = counter.getOrDefault(rc, 0) + 1;
counter.put(rc, cnt);
if (cnt == 1) {
// 这里使用 right - left (right已经++了)更好
result = Math.max(result, counter.size());
}
while (left < chars.length && counter.get(rc) > 1) {
char lc = chars[left];
left++;
int nc = counter.get(lc) - 1;
if (nc == 0) {
counter.remove(lc);
} else {
counter.put(lc, nc);
}
}
}
return result;
}