友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
402. Remove K Digits
Given a non-negative integer num represented as a string, remove k digits from the number so that the new number is the smallest possible.
Note:
-
The length of num is less than 10002 and will be ≥ k.
-
The given num does not contain any leading zero.
Example 1:
Input: num = "1432219", k = 3
Output: "1219"
Explanation: Remove the three digits 4, 3, and 2 to form the new number 1219 which is the smallest.
Example 2:
Input: num = "10200", k = 1
Output: "200"
Explanation: Remove the leading 1 and the number is 200. Note that the output must not contain leading zeroes.
Example 3:
Input: num = "10", k = 2
Output: "0"
Explanation: Remove all the digits from the number and it is left with nothing which is 0.
思路分析
利用单调栈的思路,将前面比当前字符大的字符都删除即可。
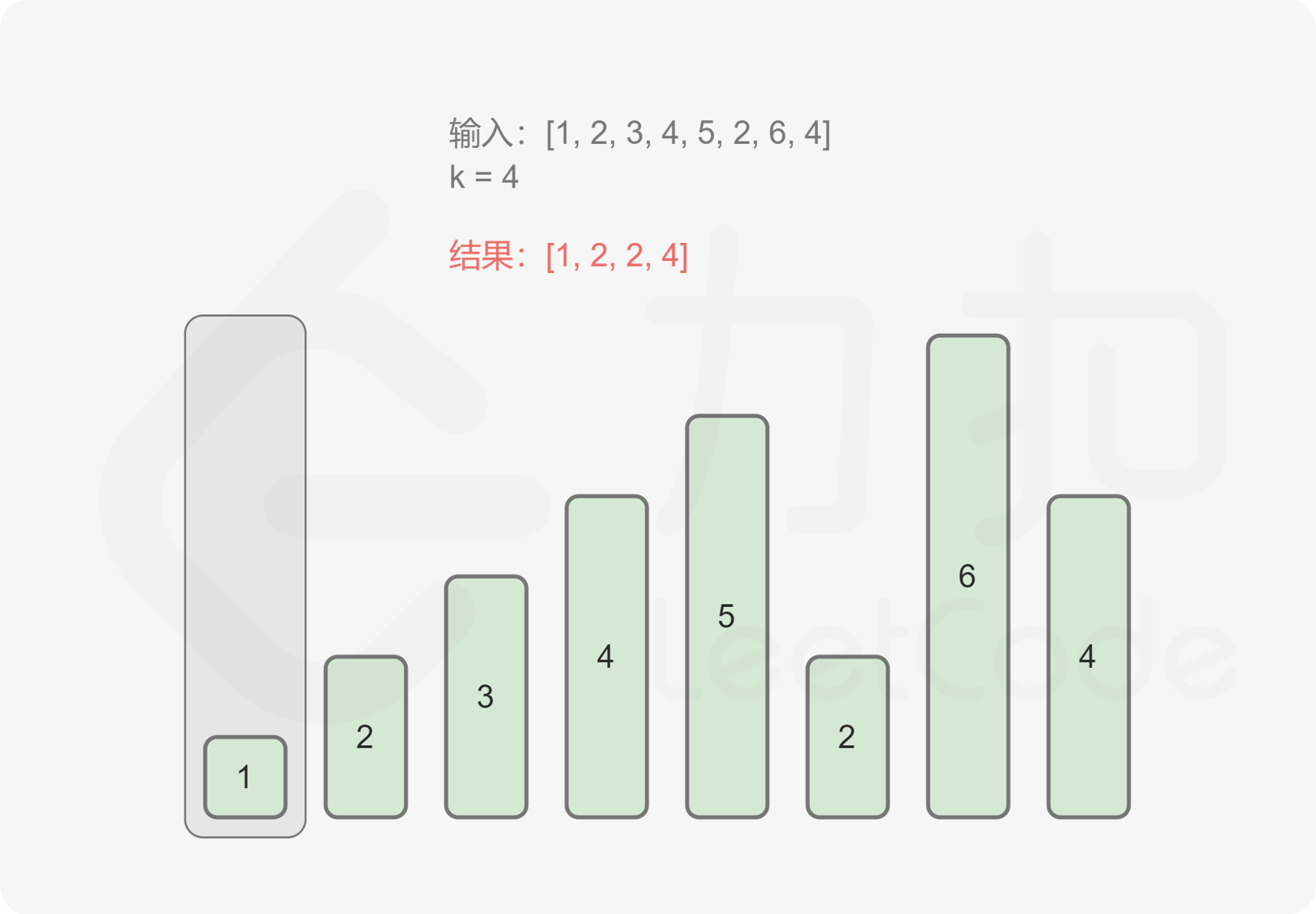
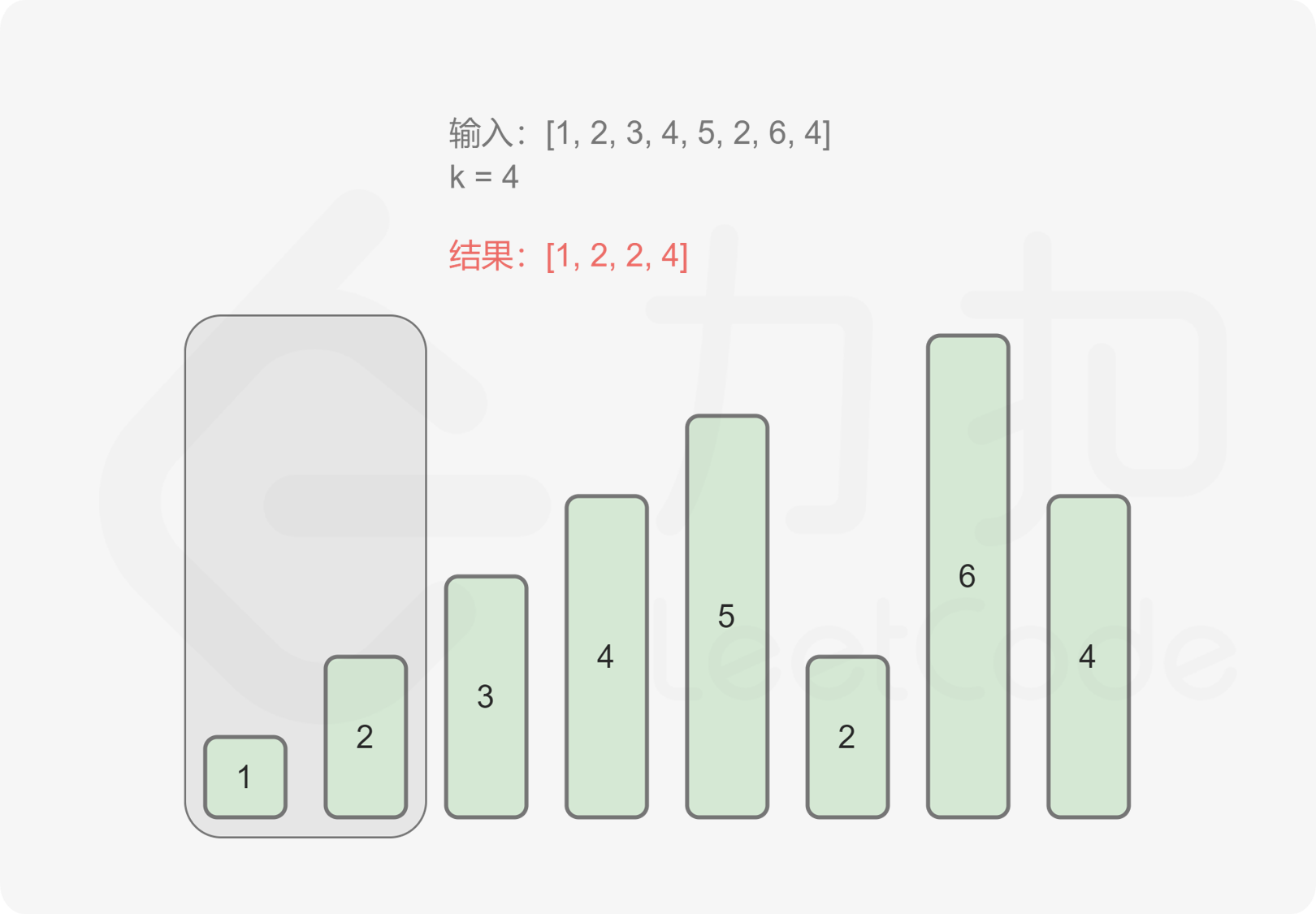
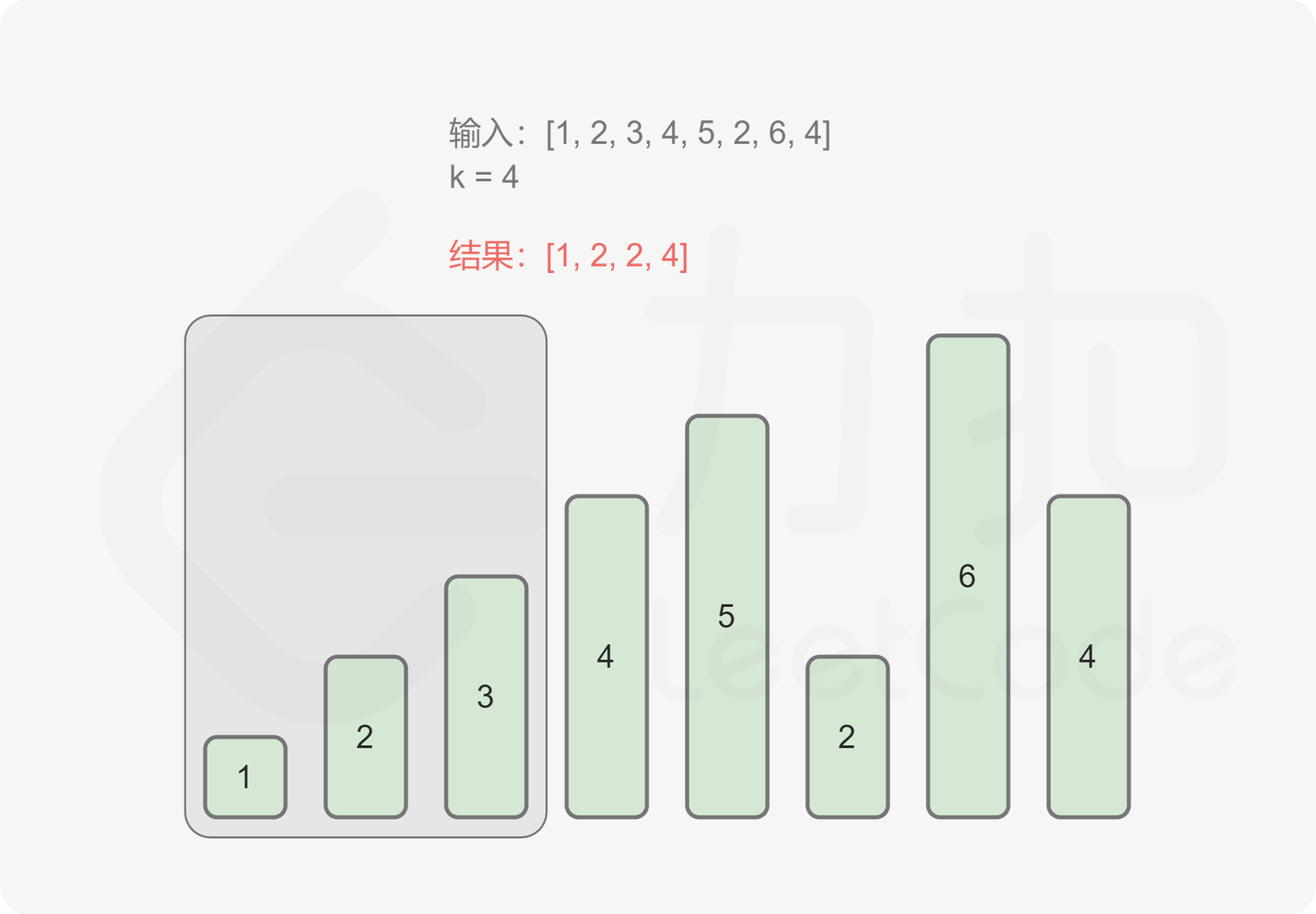
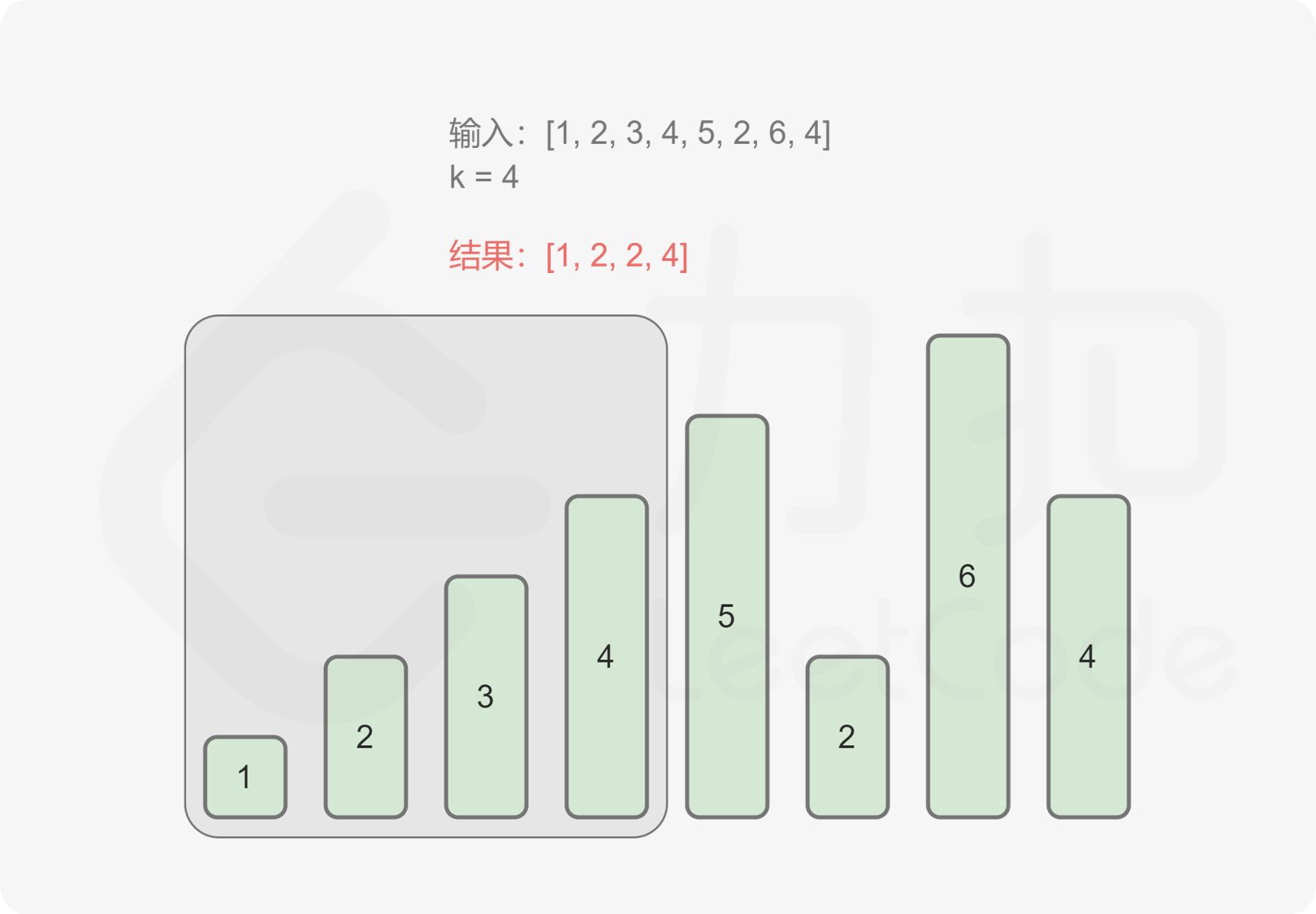
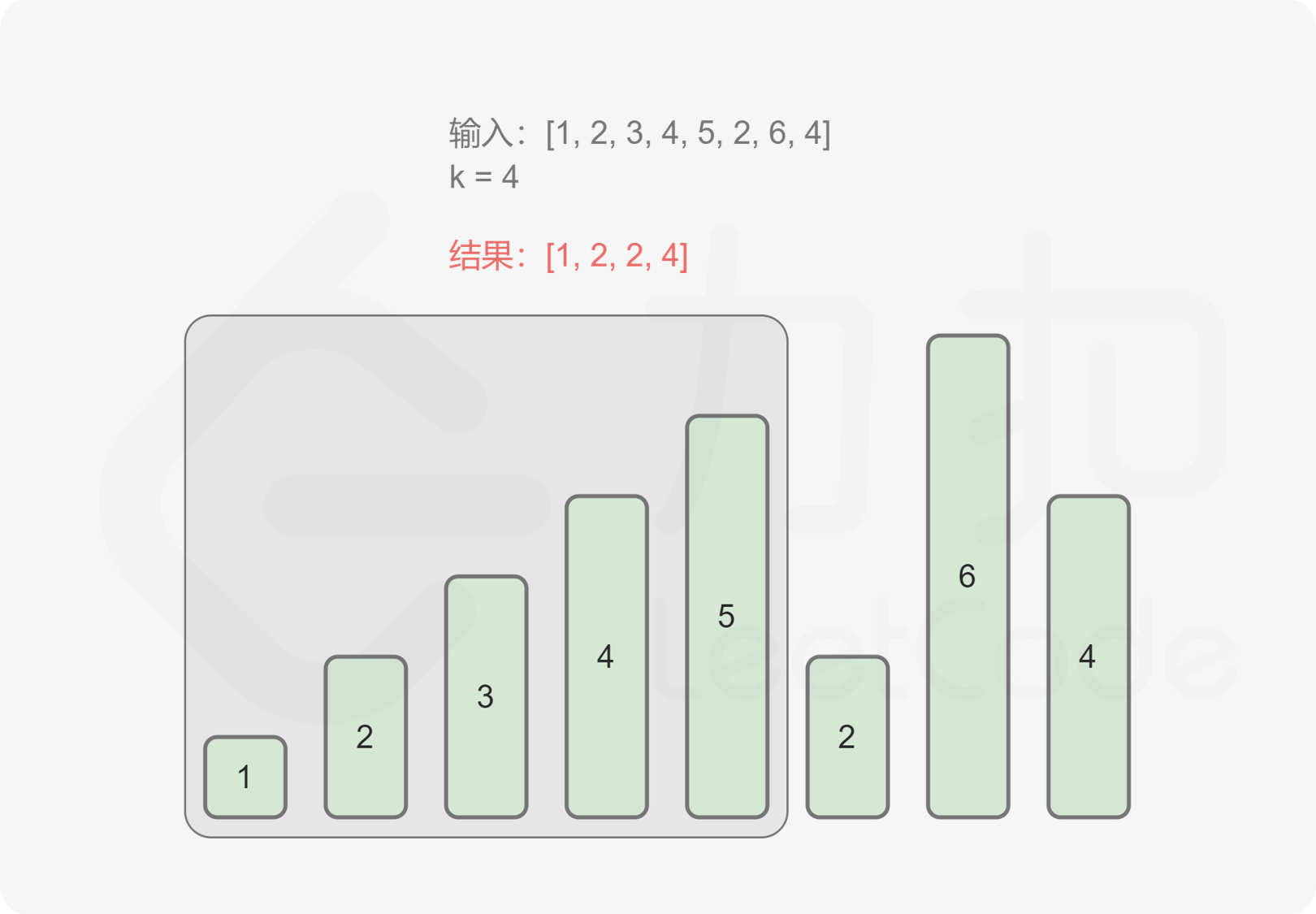
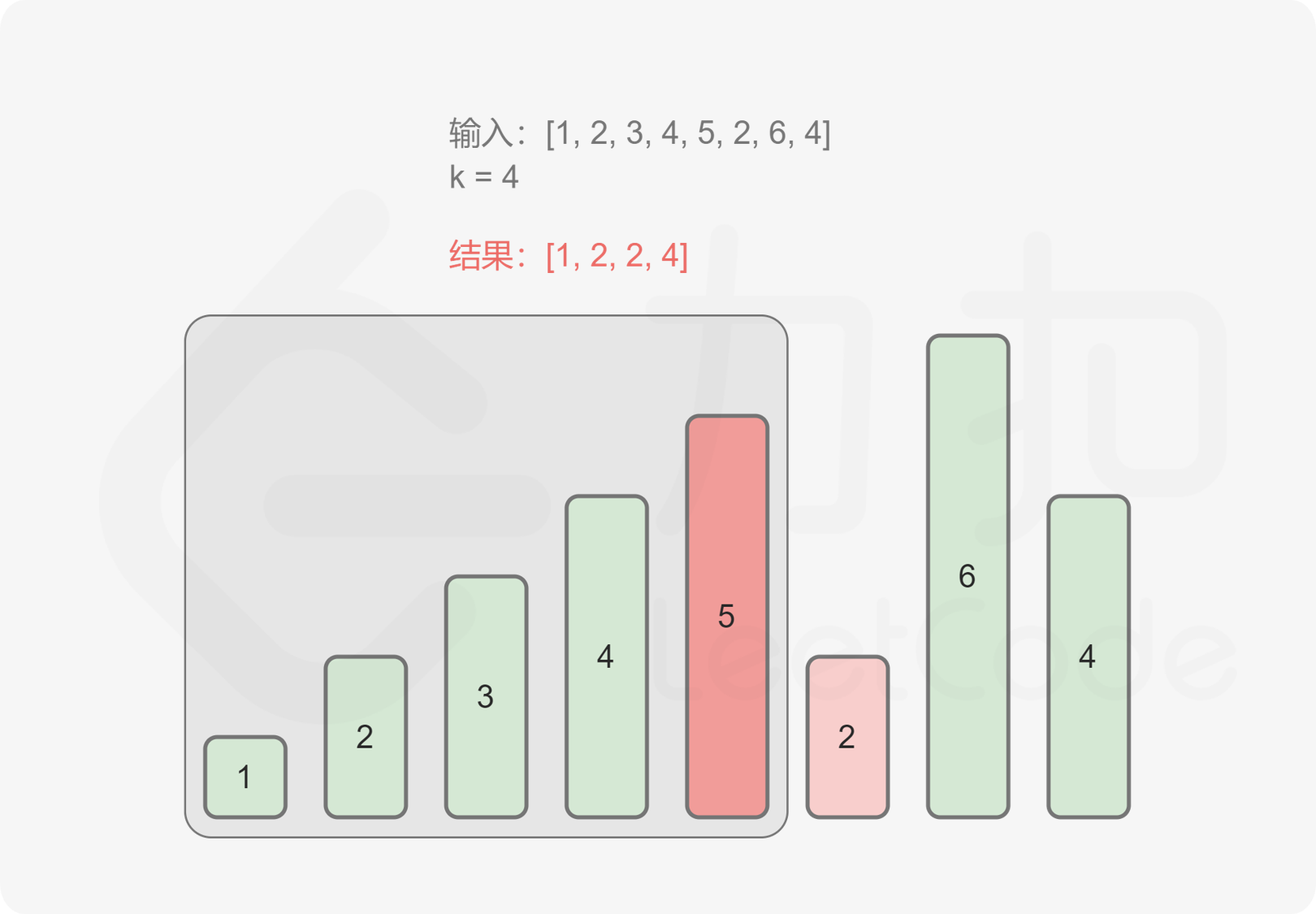
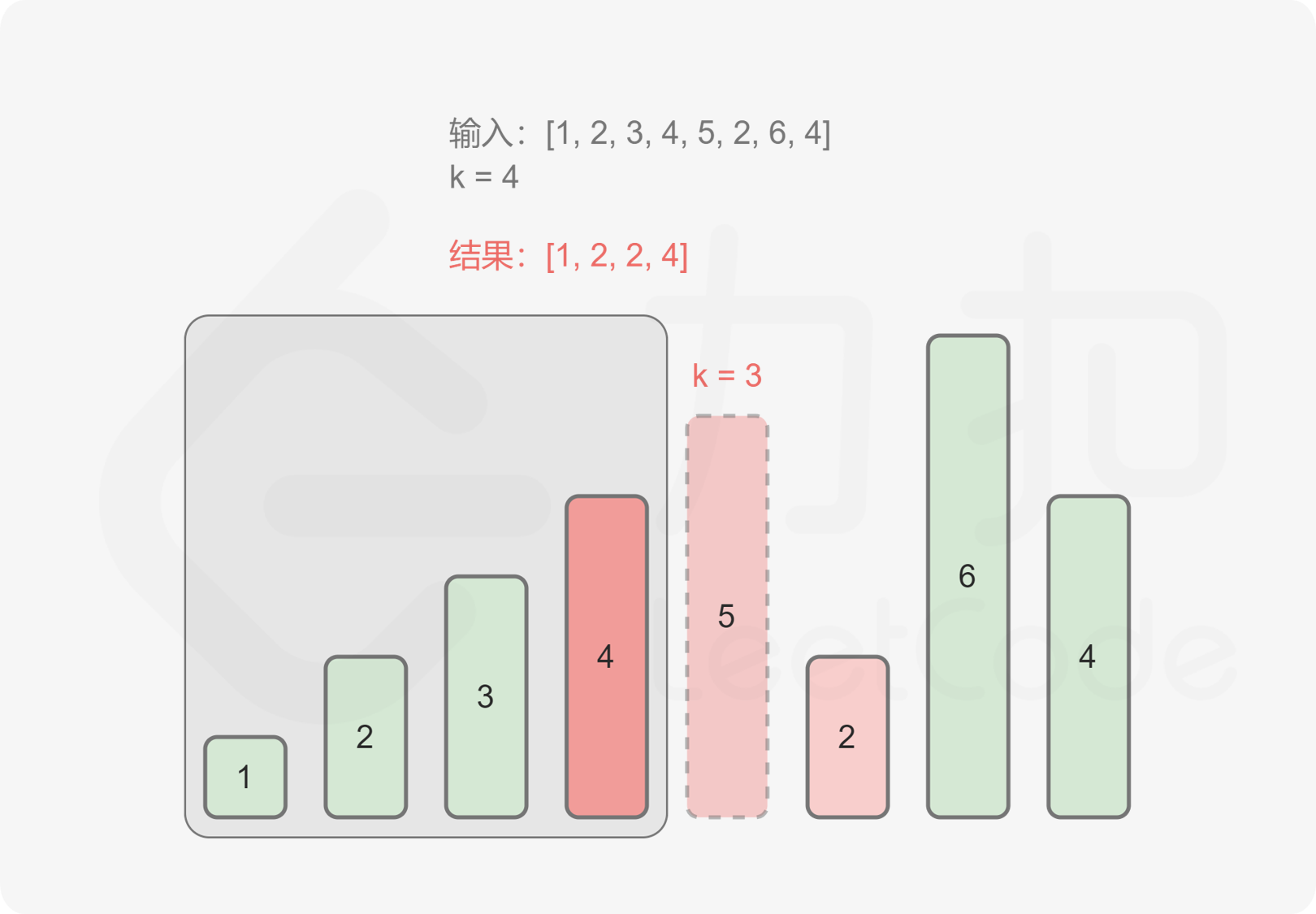
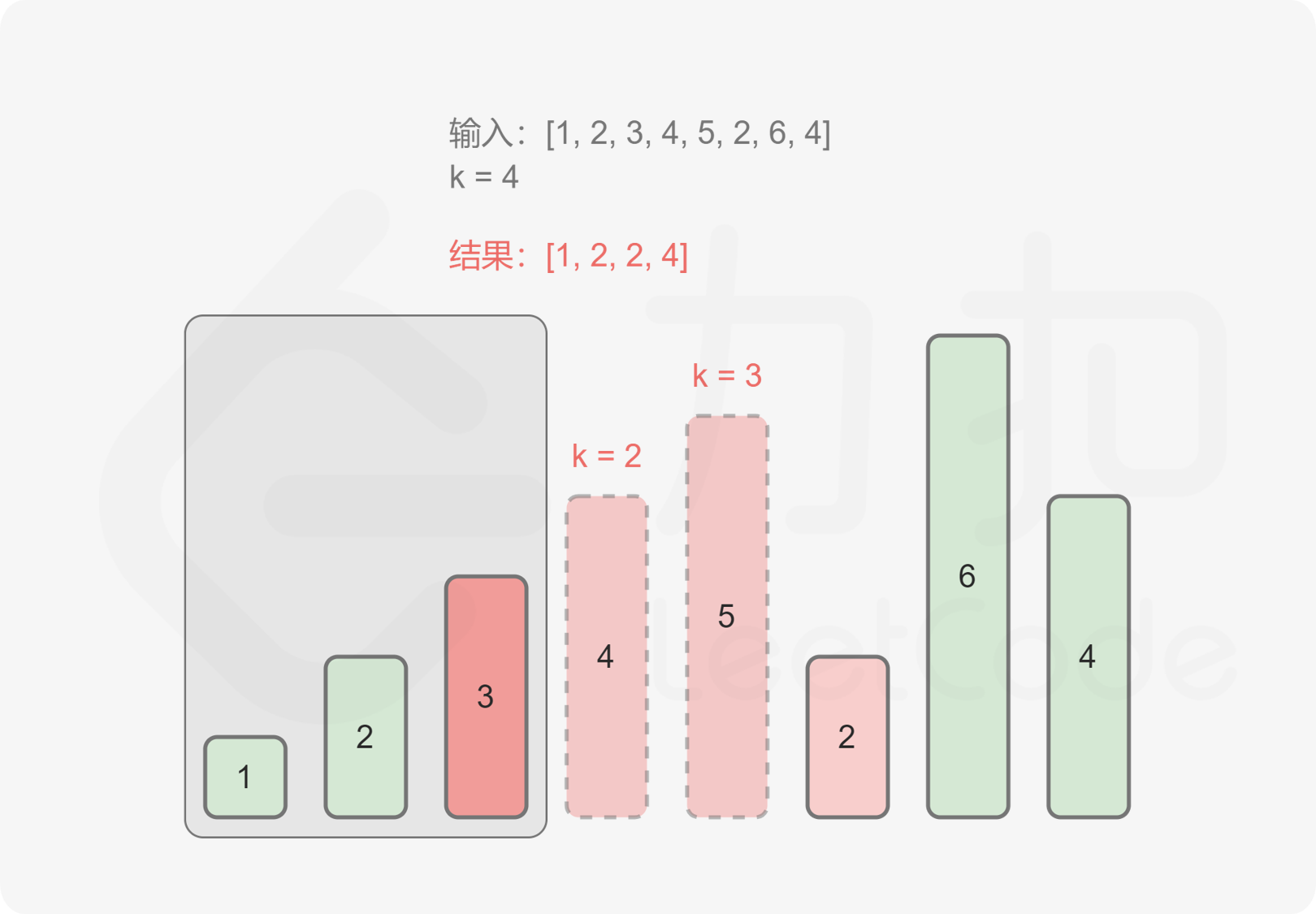
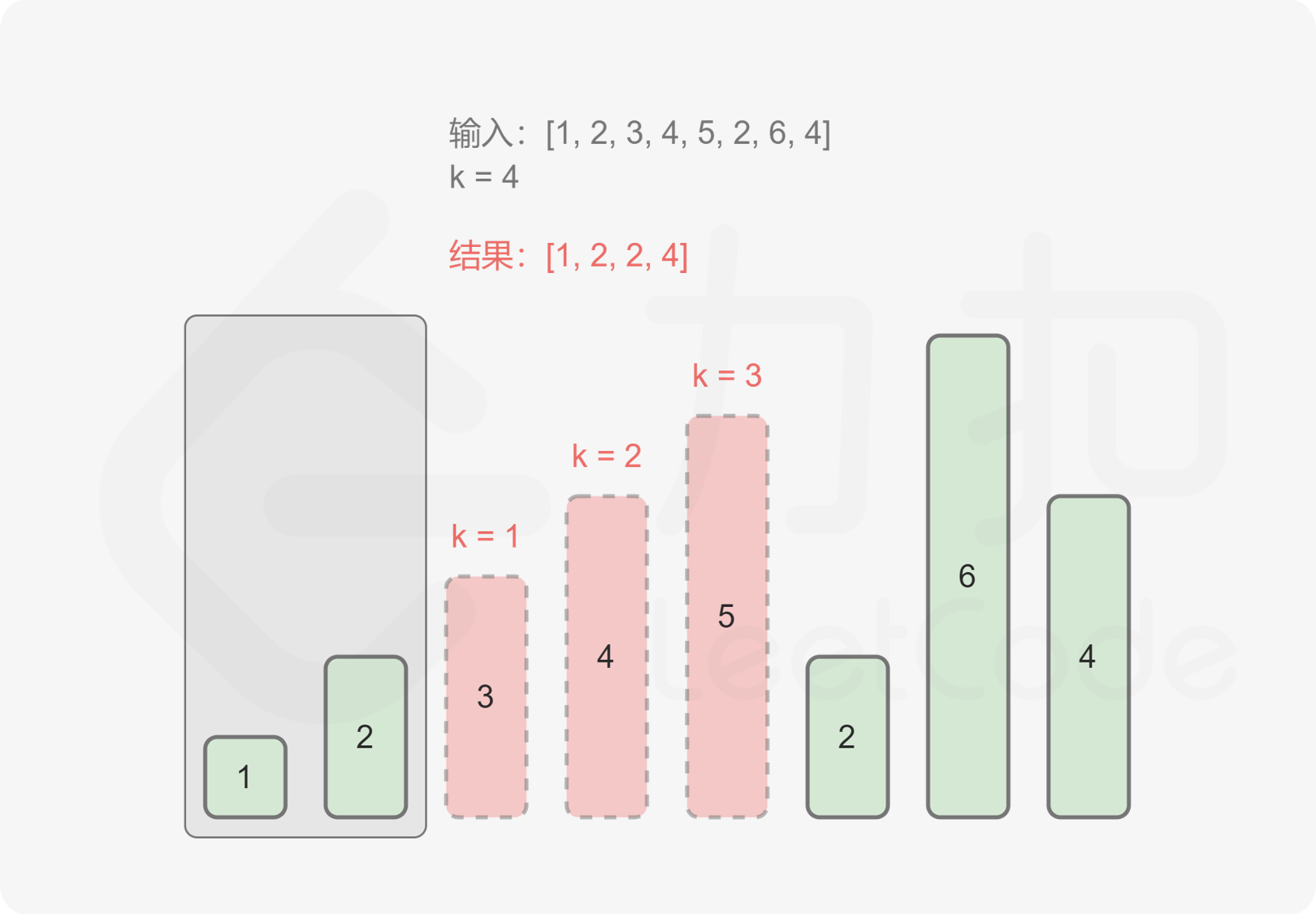
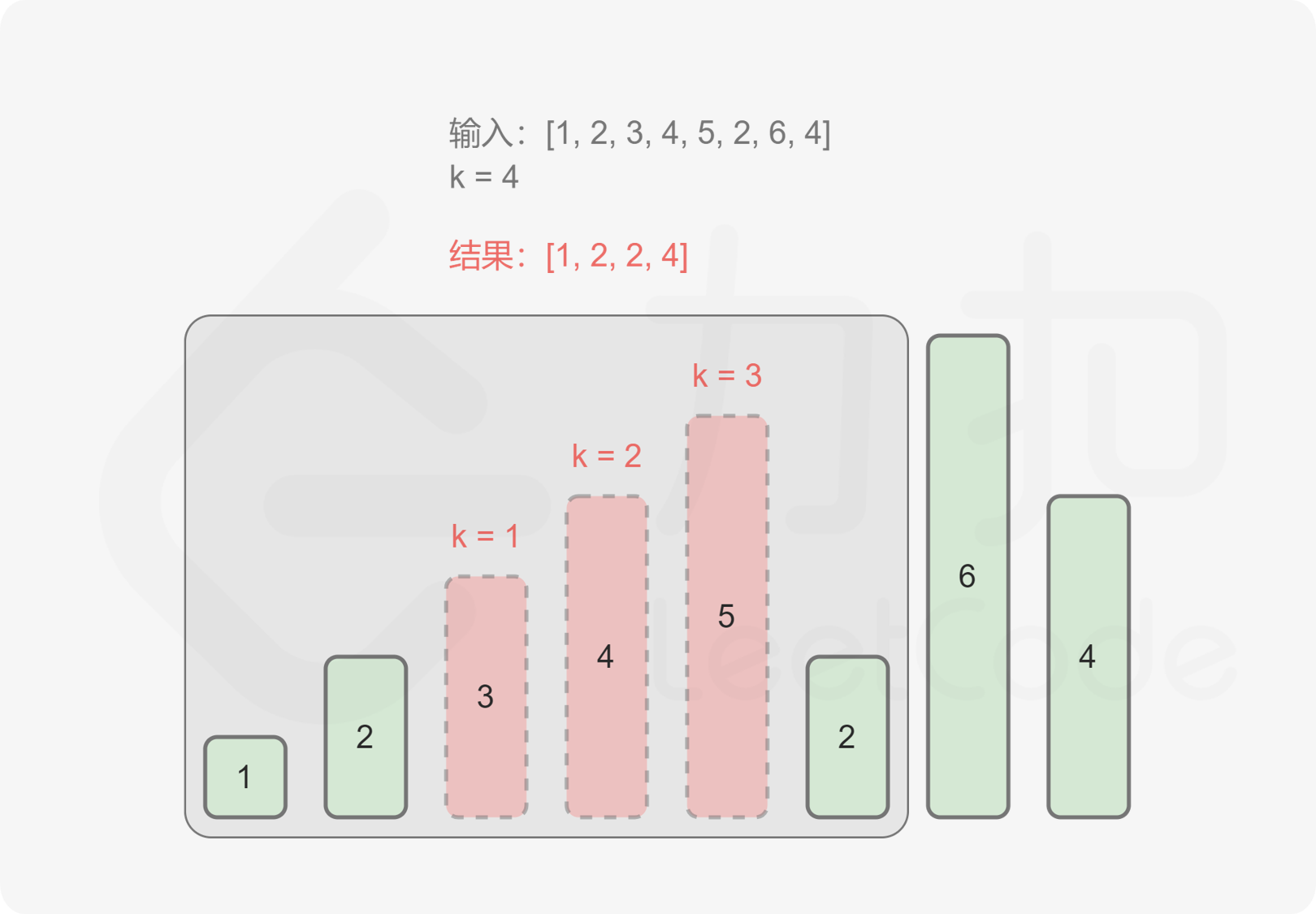
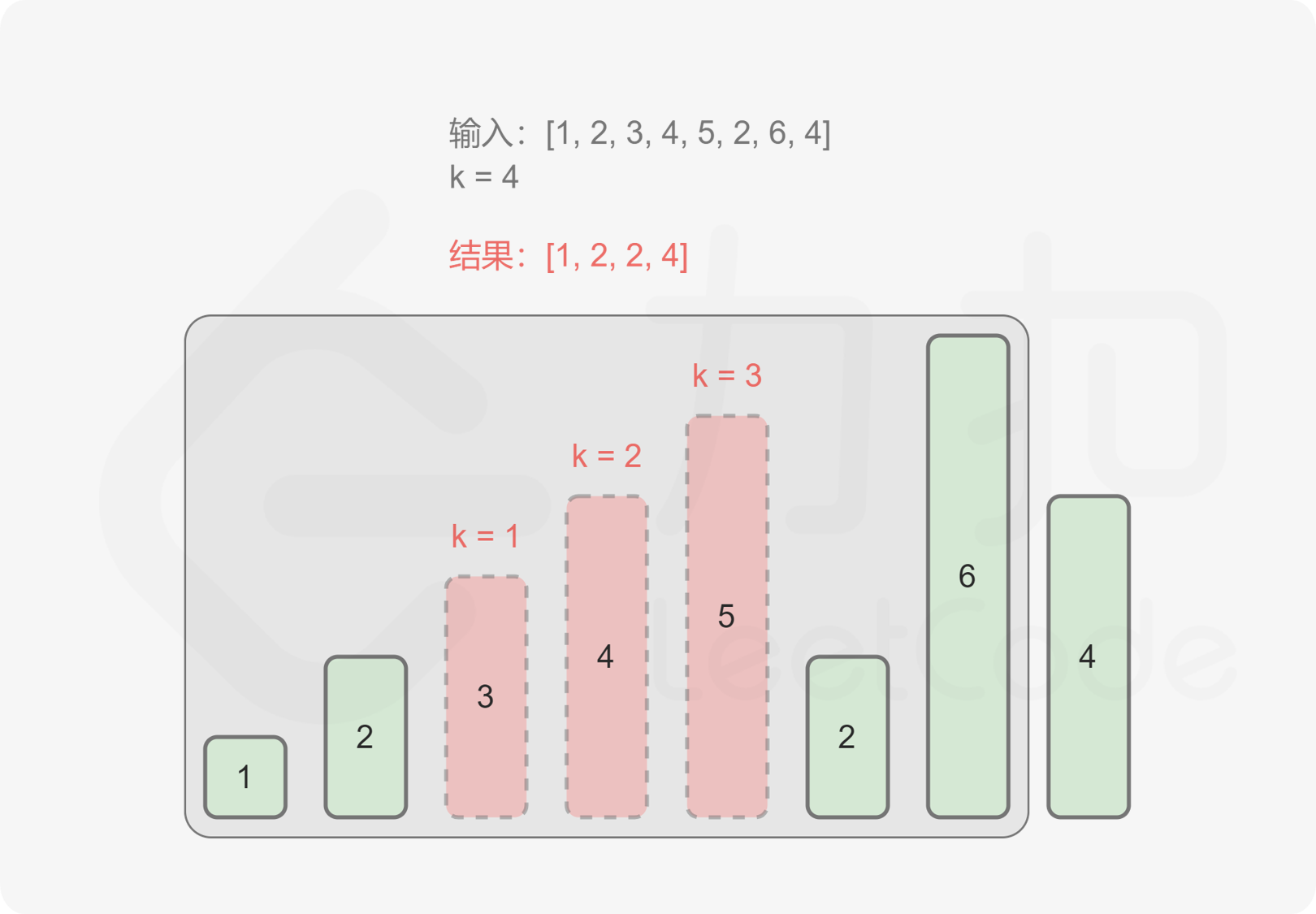
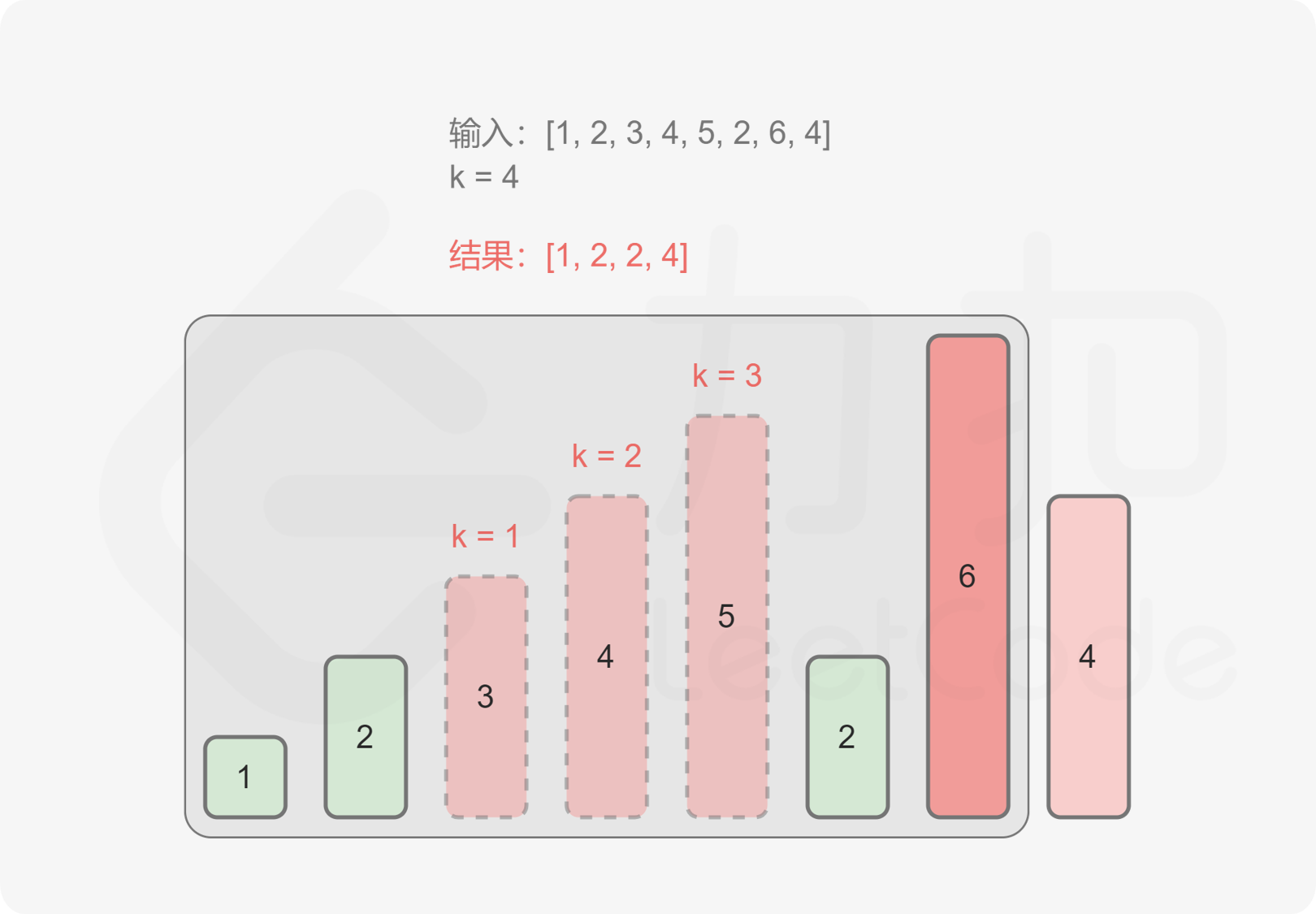
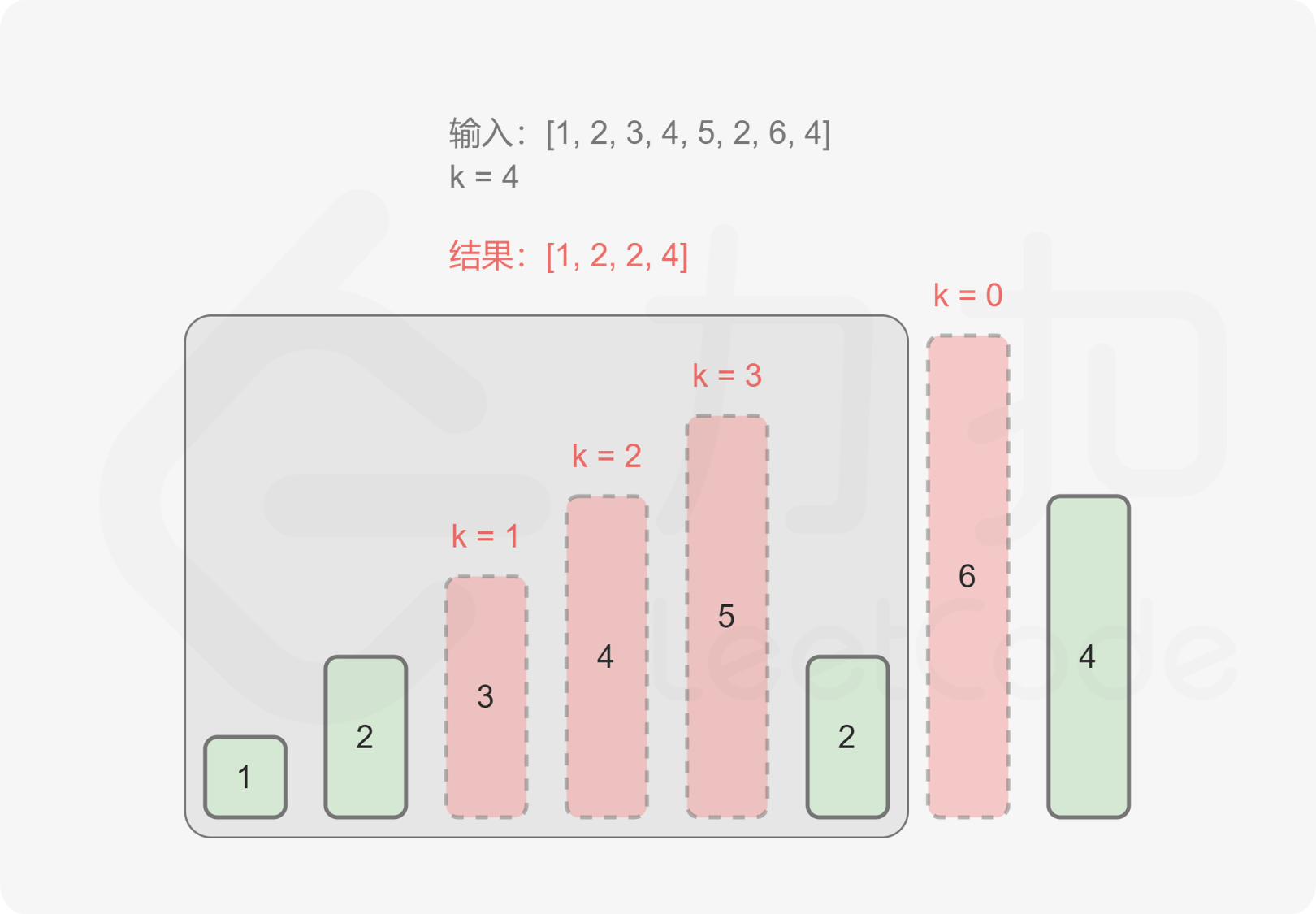
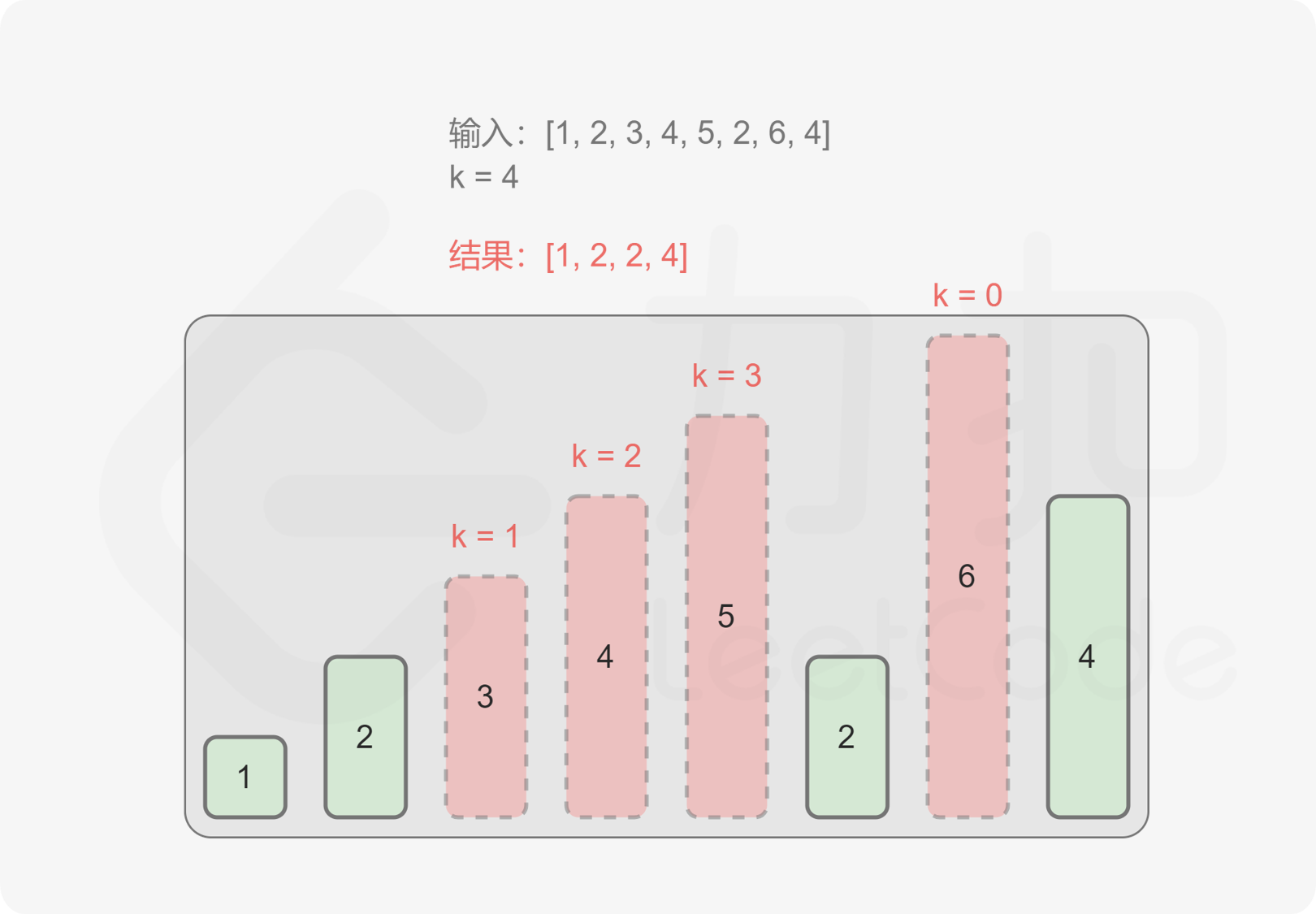
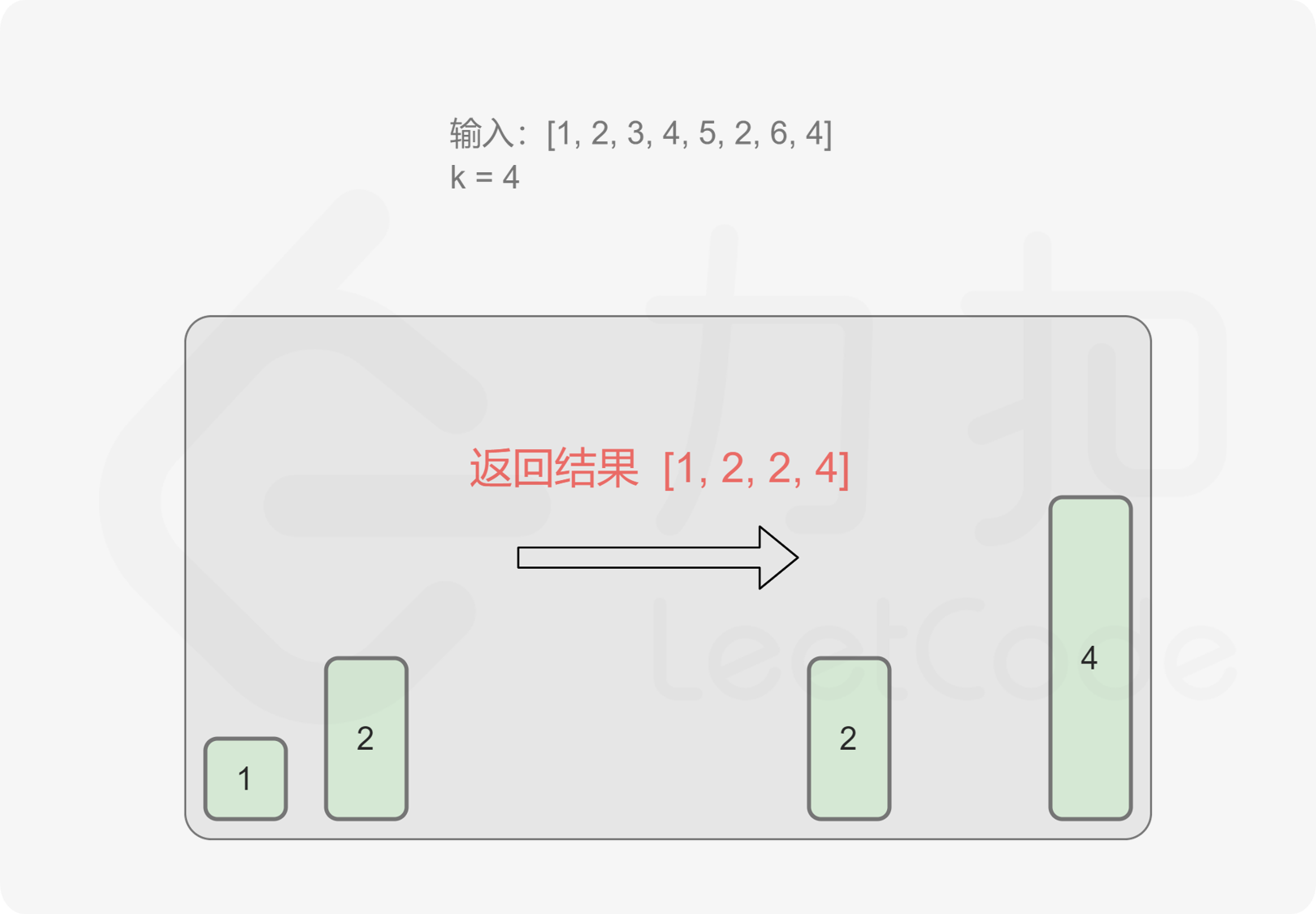
-
一刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-08-16 19:48:26
*/
public String removeKdigits(String num, int k) {
if (num == null || num.isEmpty()) {
return num;
}
if (num.length() <= k) {
return "0";
}
char[] chars = num.toCharArray();
StringBuilder stack = new StringBuilder();
stack.append(chars[0]);
for (int i = 1; i < chars.length; i++) {
char c = chars[i];
while (!stack.isEmpty() && stack.charAt(stack.length() - 1) > c && k > 0) {
stack.deleteCharAt(stack.length() - 1);
k--;
}
stack.append(c);
}
for (int i = k; i > 0; i--) {
stack.deleteCharAt(stack.length() - 1);
}
while (!stack.isEmpty() && stack.charAt(0) == '0') {
stack.deleteCharAt(0);
}
return stack.isEmpty() ? "0" : stack.toString();
}