友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
1094. Car Pooling
You are driving a vehicle that has capacity
empty seats initially available for passengers. The vehicle only drives east (ie. it cannot turn around and drive west.)
Given a list of trips
, trip[i] = [num_passengers, start_location, end_location]
contains information about the i
-th trip: the number of passengers that must be picked up, and the locations to pick them up and drop them off. The locations are given as the number of kilometers due east from your vehicle’s initial location.
Return true
if and only if it is possible to pick up and drop off all passengers for all the given trips.
Example 1:
Input: trips = [[2,1,5],[3,3,7]], capacity = 4
Output: false
Example 2:
Input: trips = [[2,1,5],[3,3,7]], capacity = 5
Output: true
Example 3:
Input: trips = [[2,1,5],[3,5,7]], capacity = 3
Output: true
Example 4:
Input: trips = [[3,2,7],[3,7,9],[8,3,9]], capacity = 11
Output: true
Constraints:
-
trips.length ⇐ 1000
-
trips[i].length == 3
-
1 ⇐ trips[i][0] ⇐ 100
-
0 ⇐ trips[i][1] < trips[i][2] ⇐ 1000
-
1 ⇐ capacity ⇐ 100000
思路分析
与 370. Range Addition 思路一样,都是差分数组。
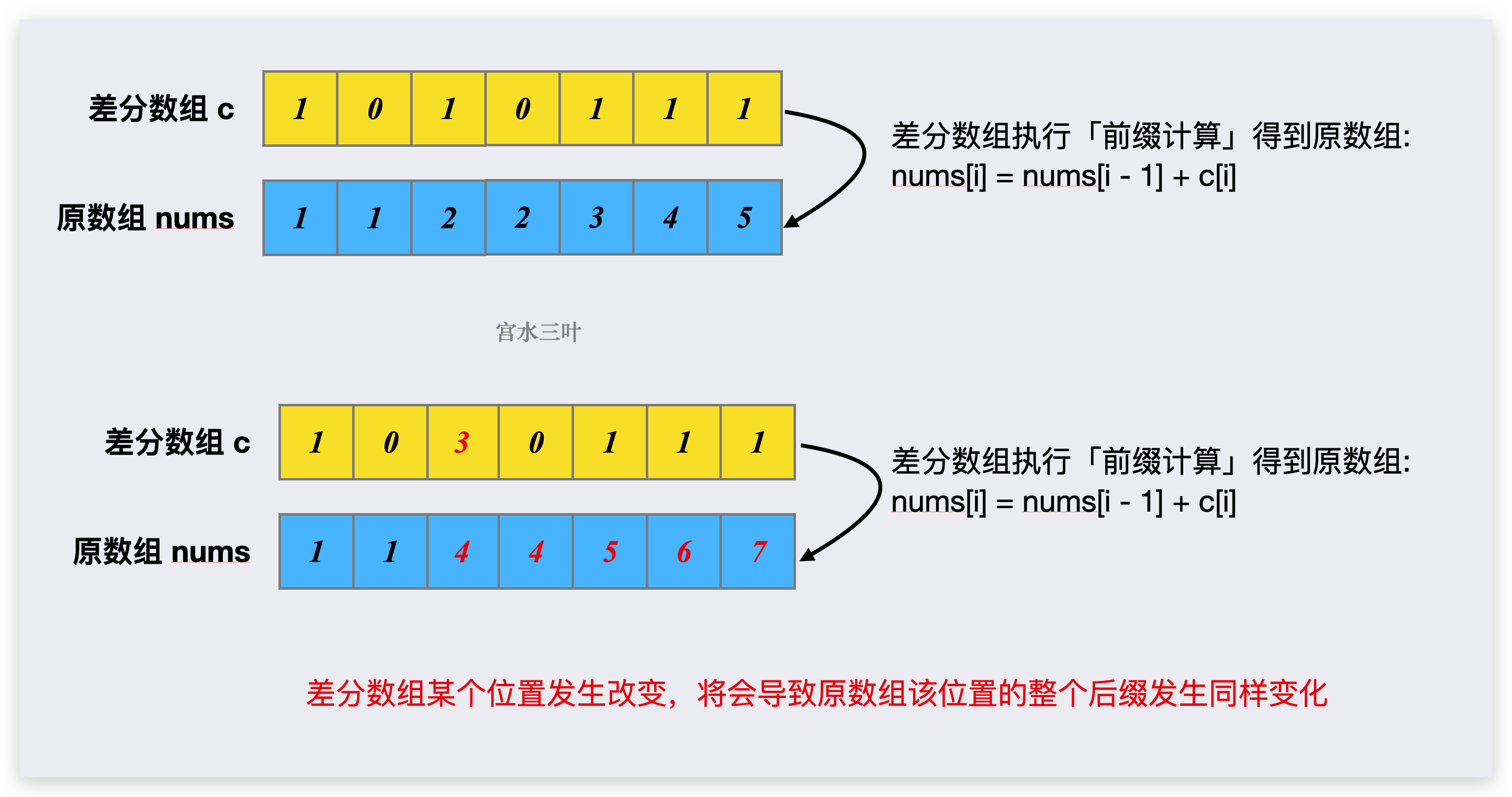
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-07-05 15:01:49
*/
public boolean carPooling(int[][] trips, int capacity) {
if (trips == null || trips.length == 0) {
return true;
}
int length = Integer.MIN_VALUE;
for (int[] trip : trips) {
if (trip[0] > capacity) {
return false;
}
length = Math.max(length, trip[2]);
}
// 差分数组
int[] diff = new int[length + 1];
for (int[] trip : trips) {
int cap = trip[0];
int start = trip[1];
int end = trip[2];
diff[start] += cap;
if (diff[start] > capacity) {
return false;
}
diff[end] -= cap;
}
for (int i = 1; i < diff.length; i++) {
diff[i] += diff[i - 1];
if (diff[i] > capacity) {
return false;
}
}
return true;
}