友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
226. Invert Binary Tree
Invert a binary tree.
Example:
Input:
4
/ \
2 7
/ \ / \
1 3 6 9
Output:
4
/ \
7 2
/ \ / \
9 6 3 1
Trivia:
This problem was inspired by this original tweet by Max Howell (@mxcl):
思路分析
D瓜哥也不会做这道题,现在可以说跟大神一个水平了,😆
其实,思路很简单:就是递归地反转每棵树即可。
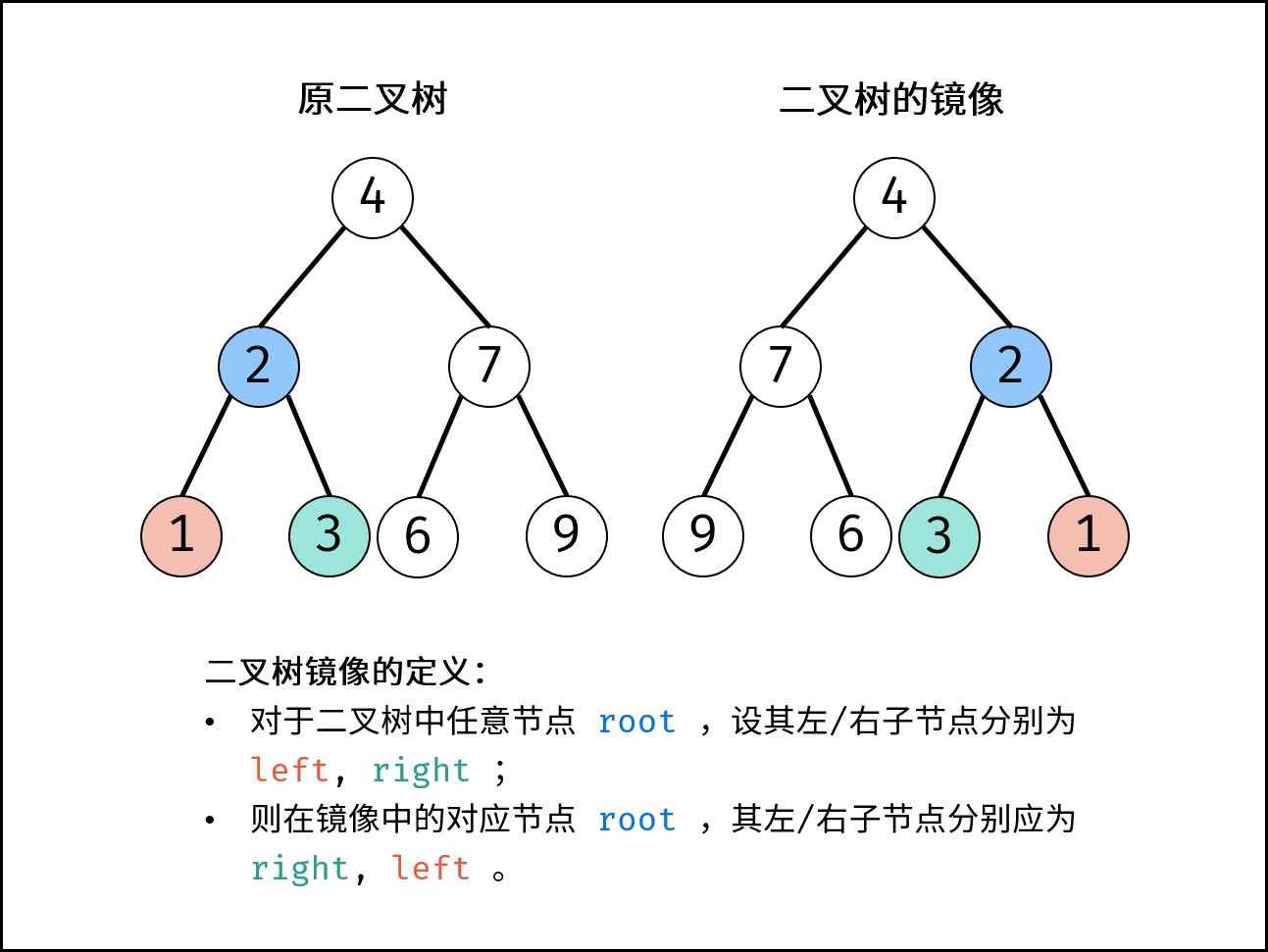
-
一刷
-
二刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Invert Binary Tree.
* Memory Usage: 37.1 MB, less than 5.10% of Java online submissions for Invert Binary Tree.
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-01-28 11:33
*/
public TreeNode invertTree(TreeNode root) {
if (Objects.isNull(root)) {
return null;
}
TreeNode left = invertTree(root.left);
TreeNode right = invertTree(root.right);
root.right = left;
root.left = right;
return root;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Invert Binary Tree.
* Memory Usage: 37.1 MB, less than 5.10% of Java online submissions for Invert Binary Tree.
*
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-13 23:03:35
*/
public TreeNode invertTree(TreeNode root) {
if (root == null) {
return root;
}
TreeNode left = invertTree(root.left);
TreeNode right = invertTree(root.right);
root.left = right;
root.right = left;
return root;
}