友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
189. 轮转数组
给定一个整数数组 nums
,将数组中的元素向右轮转 k
个位置,其中 k
是非负数。
示例 1:
输入: nums = [1,2,3,4,5,6,7], k = 3 输出: [5,6,7,1,2,3,4] 解释: 向右轮转 1 步: [7,1,2,3,4,5,6] 向右轮转 2 步: [6,7,1,2,3,4,5] 向右轮转 3 步: [5,6,7,1,2,3,4]
示例 2:
输入:nums = [-1,-100,3,99], k = 2 输出:[3,99,-1,-100] 解释: 向右轮转 1 步: [99,-1,-100,3] 向右轮转 2 步: [3,99,-1,-100]
提示:
-
1 <= nums.length <= 105
-
-231 <= nums[i] <= 231 - 1
-
0 <= k <= 105
进阶:
-
尽可能想出更多的解决方案,至少有 三种 不同的方法可以解决这个问题。
-
你可以使用空间复杂度为 \(O(1)\) 的 原地 算法解决这个问题吗?
思路分析
注意:这里是轮转数组!如果 k > nums.length
,那么轮转 k % nums.length
次和轮转 k
次的效果是一样的。
整个过程如下:
AB → rev(B)rev(A) → rev(rev(B))rev(rev(A)) → BA
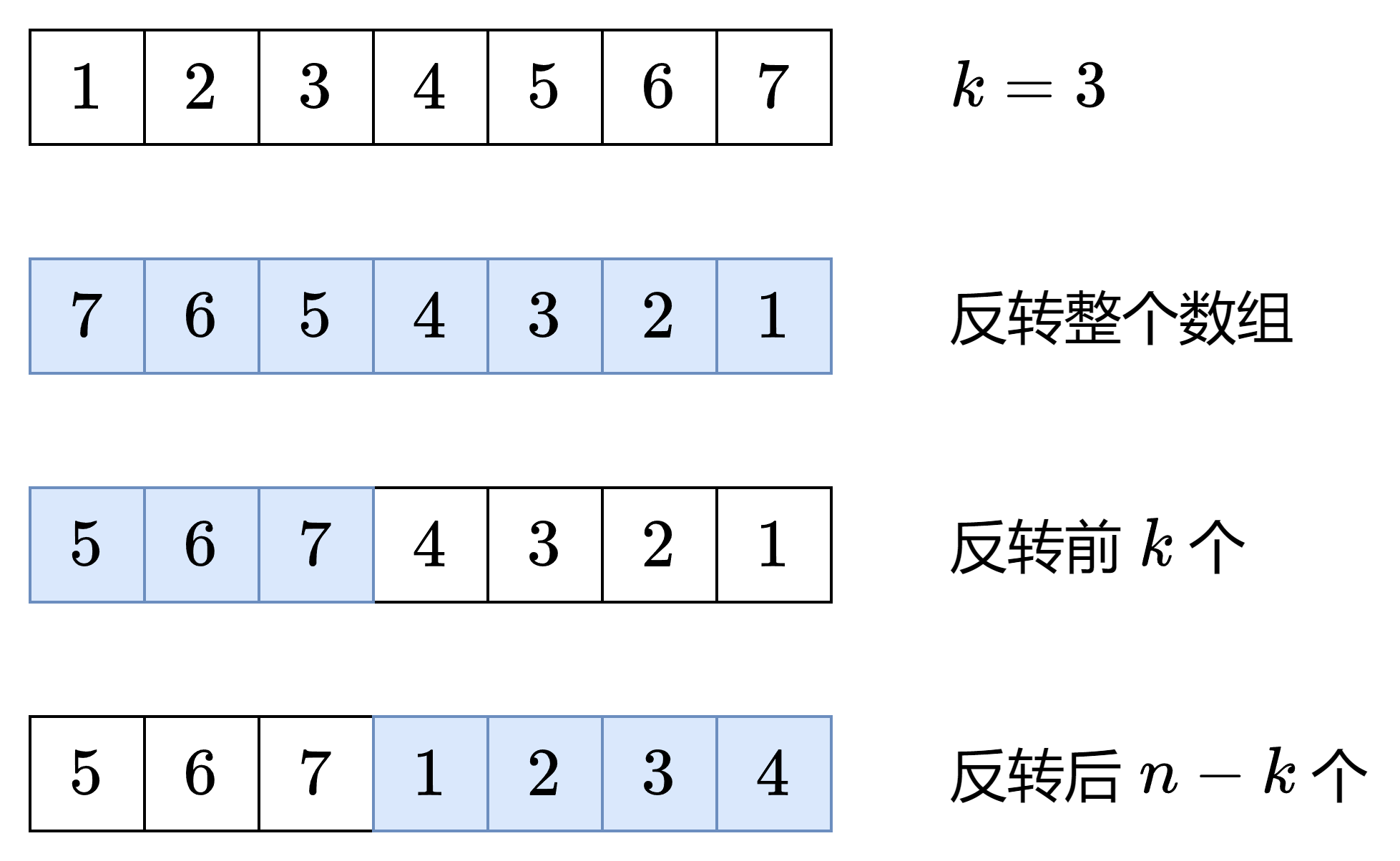
-
一刷
-
二刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Rotate Array.
*
* Memory Usage: 37.4 MB, less than 100.00% of Java online submissions for Rotate Array.
*
* Copy from: https://leetcode.com/problems/rotate-array/solution/[Rotate Array solution - LeetCode]
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-01-05 13:05
*/
public void rotate(int[] nums, int k) {
if (Objects.isNull(nums) || nums.length == 0 || k % nums.length == 0) {
return;
}
k %= nums.length;
reverse(nums, 0, nums.length - 1);
reverse(nums, 0, k - 1);
reverse(nums, k, nums.length - 1);
}
private void reverse(int[] nums, int start, int end) {
while (start < end) {
int temp = nums[start];
nums[start] = nums[end];
nums[end] = temp;
start++;
end--;
}
}
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Rotate Array.
* <p>
* Memory Usage: 38 MB, less than 85.58% of Java online submissions for Rotate Array.
*/
public void rotateExtraArray(int[] nums, int k) {
if (Objects.isNull(nums) || nums.length == 0 || k % nums.length == 0) {
return;
}
k %= nums.length;
int[] temp = new int[k];
for (int i = 0; i < k; i++) {
temp[k - i - 1] = nums[nums.length - i - 1];
}
for (int i = nums.length - 1; i >= k; i--) {
nums[i] = nums[i - k];
}
for (int i = 0; i < k; i++) {
nums[i] = temp[i];
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
/**
* AB → rev(B)rev(A) → rev(rev(B))rev(rev(A)) → BA
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-01-05 13:05
*/
public void rotate(int[] nums, int k) {
int len = nums.length;
k %= len;
if (k == 0) {
return;
}
reverse(nums, 0, len - 1);
reverse(nums, 0, k - 1);
reverse(nums, k, len - 1);
}
private void reverse(int[] nums, int start, int end) {
while (start < end) {
int temp = nums[start];
nums[start] = nums[end];
nums[end] = temp;
start++;
end--;
}
}
发现官网测试用例少了一个特殊情况:如果 k
大于两倍数组长度,提交的测试就会报错,但是官网却显示通过。
数组反转的方法也不错!反转时,直接使用界限的值进行加减比较简单省事!
参考资料
-
189. 轮转数组 - 官方题解 — 环状替换有意思!