友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
61. Rotate List
Given a linked list, rotate the list to the right by k
places, where k is non-negative.
Example 1:
Input: 1->2->3->4->5->NULL, k = 2 Output: 4->5->1->2->3->NULL Explanation: rotate 1 steps to the right: 5->1->2->3->4->NULL rotate 2 steps to the right: 4->5->1->2->3->NULL
Example 2:
Input: 0->1->2->NULL, k = 4 Output: 2->0->1->NULL Explanation: rotate 1 steps to the right: 2->0->1->NULL rotate 2 steps to the right: 1->2->0->NULL rotate 3 steps to the right: 0->1->2->NULL rotate 4 steps to the right: 2->0->1->NULL
解题分析
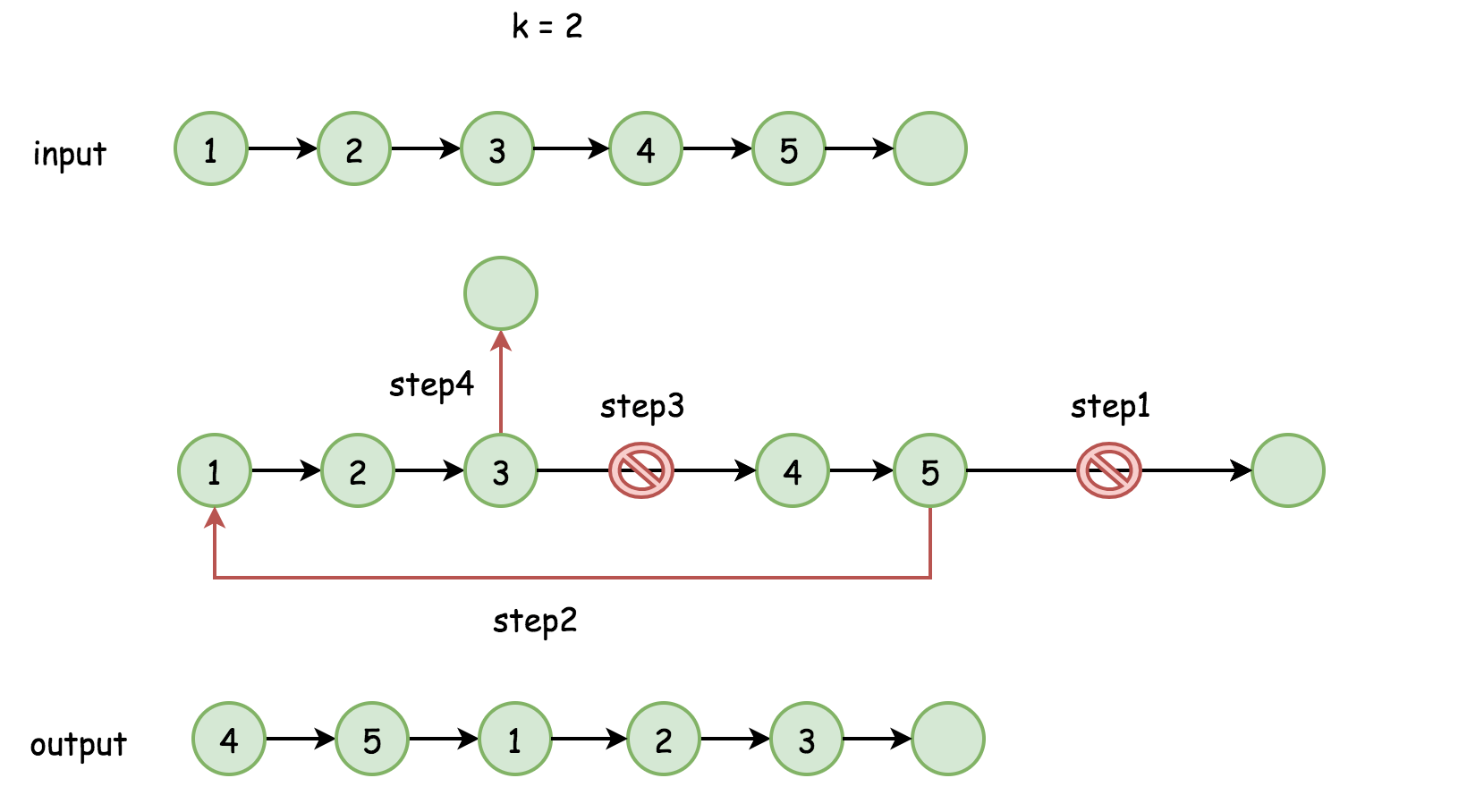
这个环形解题法很赞!
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Rotate List.
* Memory Usage: 38.8 MB, less than 46.55% of Java online submissions for Rotate List.
*
* Copy from: https://leetcode-cn.com/problems/rotate-list/solution/xuan-zhuan-lian-biao-by-leetcode/[旋转链表 - 旋转链表 - 力扣(LeetCode)]
*
* @author D瓜哥 · https://www.diguage.com
* @since 2020-02-01 23:11
*/
public ListNode rotateRight(ListNode head, int k) {
if (Objects.isNull(head) || Objects.isNull(head.next) || k == 0) {
return head;
}
ListNode current = head;
int length;
for (length = 1; Objects.nonNull(current.next); length++) {
current = current.next;
}
int pass = length - k % length;
if (pass == length || pass == 0) {
return head;
}
current.next = head;
current = head;
for (int i = 0; i < pass - 1; i++) {
current = current.next;
}
ListNode result = current.next;
current.next = null;
return result;
}
/**
* Runtime: 0 ms, faster than 100.00% of Java online submissions for Rotate List.
* Memory Usage: 38.7 MB, less than 55.17% of Java online submissions for Rotate List.
*/
public ListNode rotateRightLoop(ListNode head, int k) {
if (Objects.isNull(head)) {
return null;
}
if (k == 0) {
return head;
}
ListNode current = head;
int length = 0;
while (Objects.nonNull(current)) {
length++;
current = current.next;
}
int pass = length - k % length;
if (pass == length || pass == 0) {
return head;
}
current = head;
for (int i = 0; i < pass - 1; i++) {
current = current.next;
}
ListNode result = current.next;
current.next = null;
current = result;
while (Objects.nonNull(current)) {
if (Objects.isNull(current.next)) {
break;
}
current = current.next;
}
current.next = head;
return result;
}