友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
13. Roman to Integer
Roman numerals are represented by seven different symbols: I
, V
, X
, L
, C
, D
and M
.
Symbol Value I 1 V 5 X 10 L 50 C 100 D 500 M 1000
For example, two is written as II
in Roman numeral, just two one’s added together. Twelve is written as, XII
, which is simply X
+ II
. The number twenty seven is written as XXVII
, which is XX
+ V
+ II
.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not IIII
. Instead, the number four is written as IV
. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX
. There are six instances where subtraction is used:
-
I
can be placed beforeV
(5) andX
(10) to make 4 and 9. -
X
can be placed beforeL
(50) andC
(100) to make 40 and 90. -
C
can be placed beforeD
(500) andM
(1000) to make 400 and 900.
Given a roman numeral, convert it to an integer. Input is guaranteed to be within the range from 1 to 3999.
Example 1:
Input: "III" Output: 3
Example 2:
Input: "IV" Output: 4
Example 3:
Input: "IX" Output: 9
Example 4:
Input: "LVIII" Output: 58 Explanation: L = 50, V= 5, III = 3.
Example 5:
Input: "MCMXCIV" Output: 1994 Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
解题分析
查看罗马数字,如果左边的数字比右边大,则就是需要做减法,否则做加法。
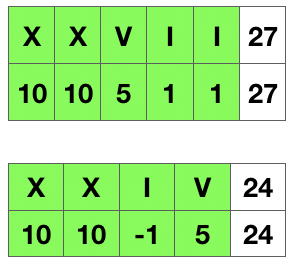
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
/**
* 从右向左,从小到大,更容易理解
*
* @author D瓜哥 · https://www.diguage.com
* @since 2019-07-11 17:14
*/
public int romanToInt(String s) {
int result = 0;
int pre = 0;
for (int i = s.length() - 1; i >= 0; i--) {
int curr = charToNum(s.charAt(i));
if (curr >= pre) {
result += curr;
} else {
result -= curr;
}
pre = curr;
}
return result;
}
private int charToNum(char c) {
switch (c) {
case 'I': return 1;
case 'V': return 5;
case 'X': return 10;
case 'L': return 50;
case 'C': return 100;
case 'D': return 500;
case 'M': return 1000;
default: return 0;
}
}
// 从左向右处理
public int romanToIntLeftToRight(String s) {
int result = 0;
int prenum = charToNum(s.charAt(0));
for (int i = 1; i < s.length(); i++) {
int num = charToNum(s.charAt(i));
if (prenum < num) {
result -= prenum;
} else {
result += prenum;
}
prenum = num;
}
result += prenum;
return result;
}
public int romanToInt2(String s) {
int result = 0;
if (Objects.isNull(s) || s.length() == 0) {
return result;
}
HashMap<String, Integer> r2i = new HashMap<>();
r2i.put("I", 1);
r2i.put("V", 5);
r2i.put("X", 10);
r2i.put("L", 50);
r2i.put("C", 100);
r2i.put("D", 500);
r2i.put("M", 1000);
r2i.put("IV", 4);
r2i.put("IX", 9);
r2i.put("XL", 40);
r2i.put("XC", 90);
r2i.put("CD", 400);
r2i.put("CM", 900);
for (int i = s.length(); i > 0; ) {
int step = 2;
int beginIndex = i - step;
if (beginIndex < 0) {
beginIndex = 0;
}
String symbol = s.substring(beginIndex, i);
Integer value = r2i.get(symbol);
if (Objects.isNull(value)) {
step = 1;
beginIndex = i - step;
if (beginIndex < 0) {
beginIndex = 0;
}
symbol = s.substring(beginIndex, i);
value = r2i.get(symbol);
}
result += value;
i -= step;
}
return result;
}