友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
39. Combination Sum
Given a set of candidate numbers (candidates
) (without duplicates) and a target number (target
), find all unique combinations in candidates
where the candidate numbers sums to target
.
The same repeated number may be chosen from candidates
unlimited number of times.
Note:
-
All numbers (including target) will be positive integers.
-
The solution set must not contain duplicate combinations.
Example 1:
Input: candidates = [2,3,6,7], target = 7,
A solution set is:
[
[7],
[2,2,3]
]
Example 2:
Input: candidates = [2,3,5], target = 8,
A solution set is:
[
[2,2,2,2],
[2,3,3],
[3,5]
]
思路分析
参考 46. Permutations 认真学习一下回溯思想。
为了避免重复,每次只遍历不比自己小的元素。(比自己小的元素已经被小元素遍历过了。)
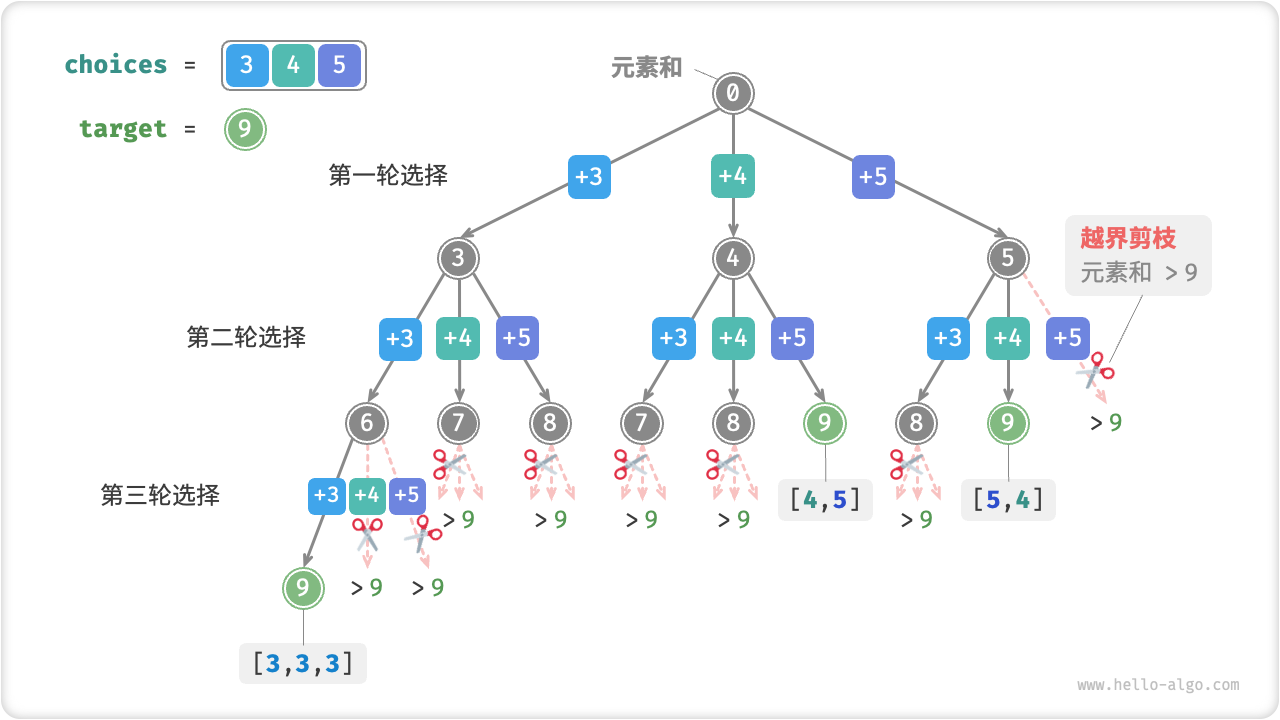
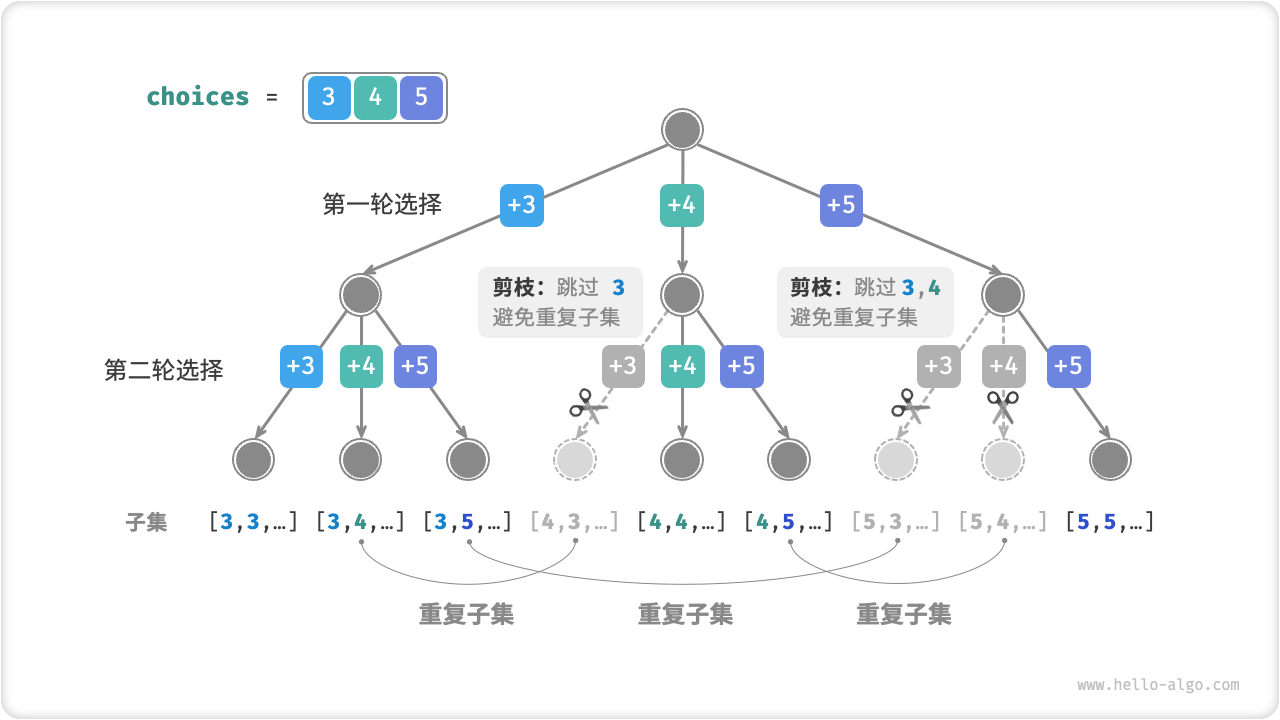
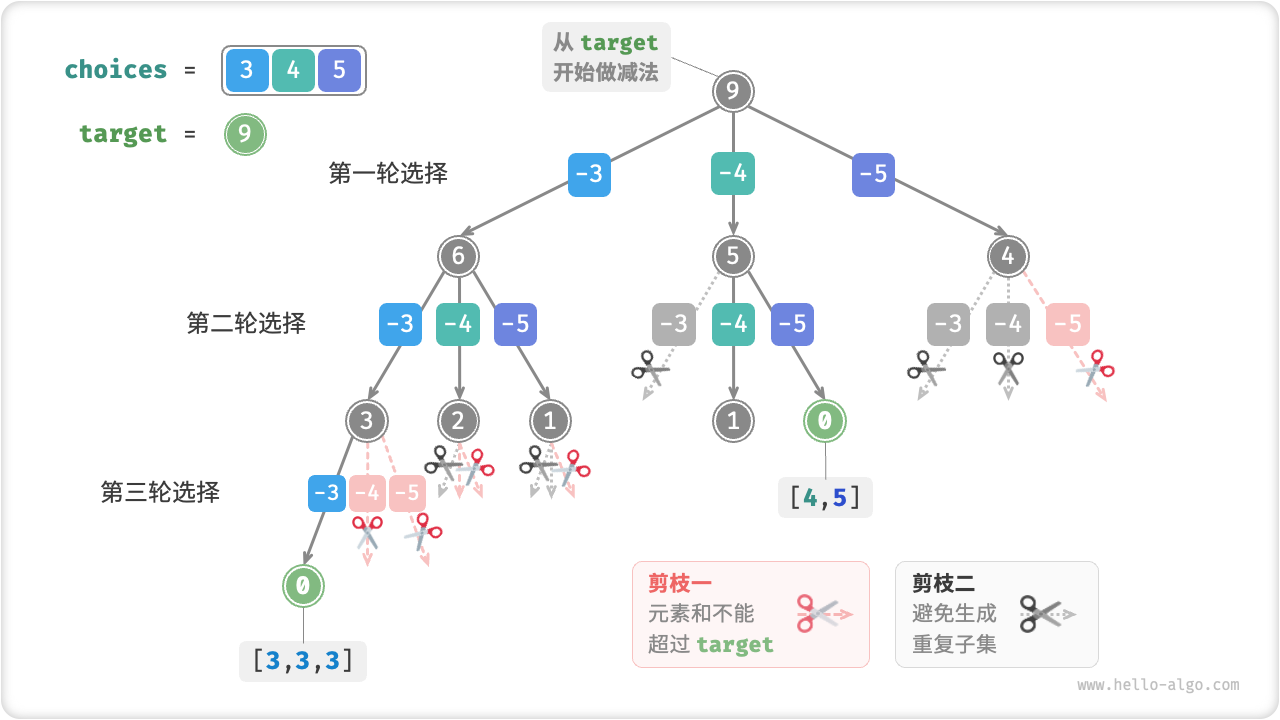
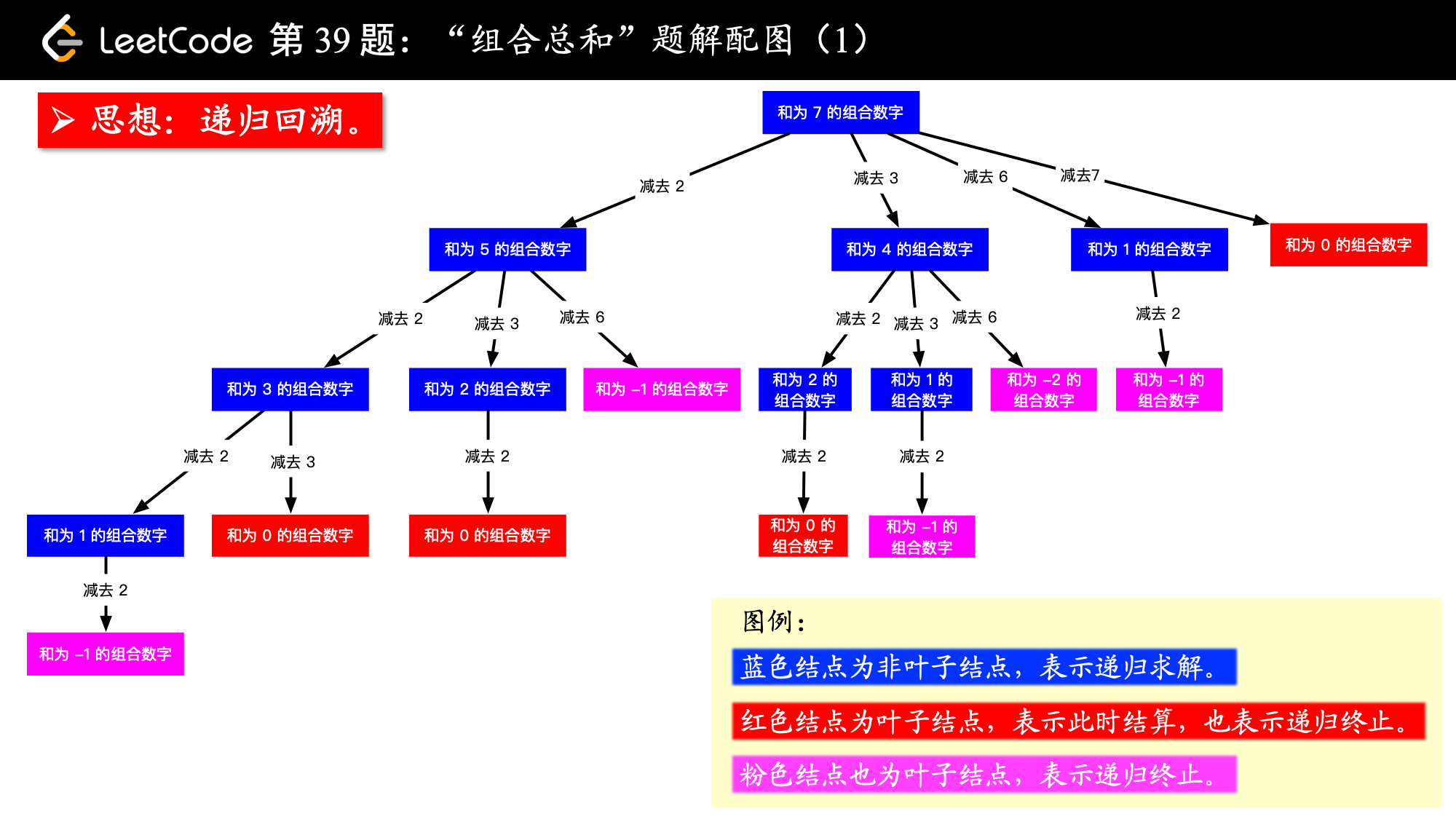
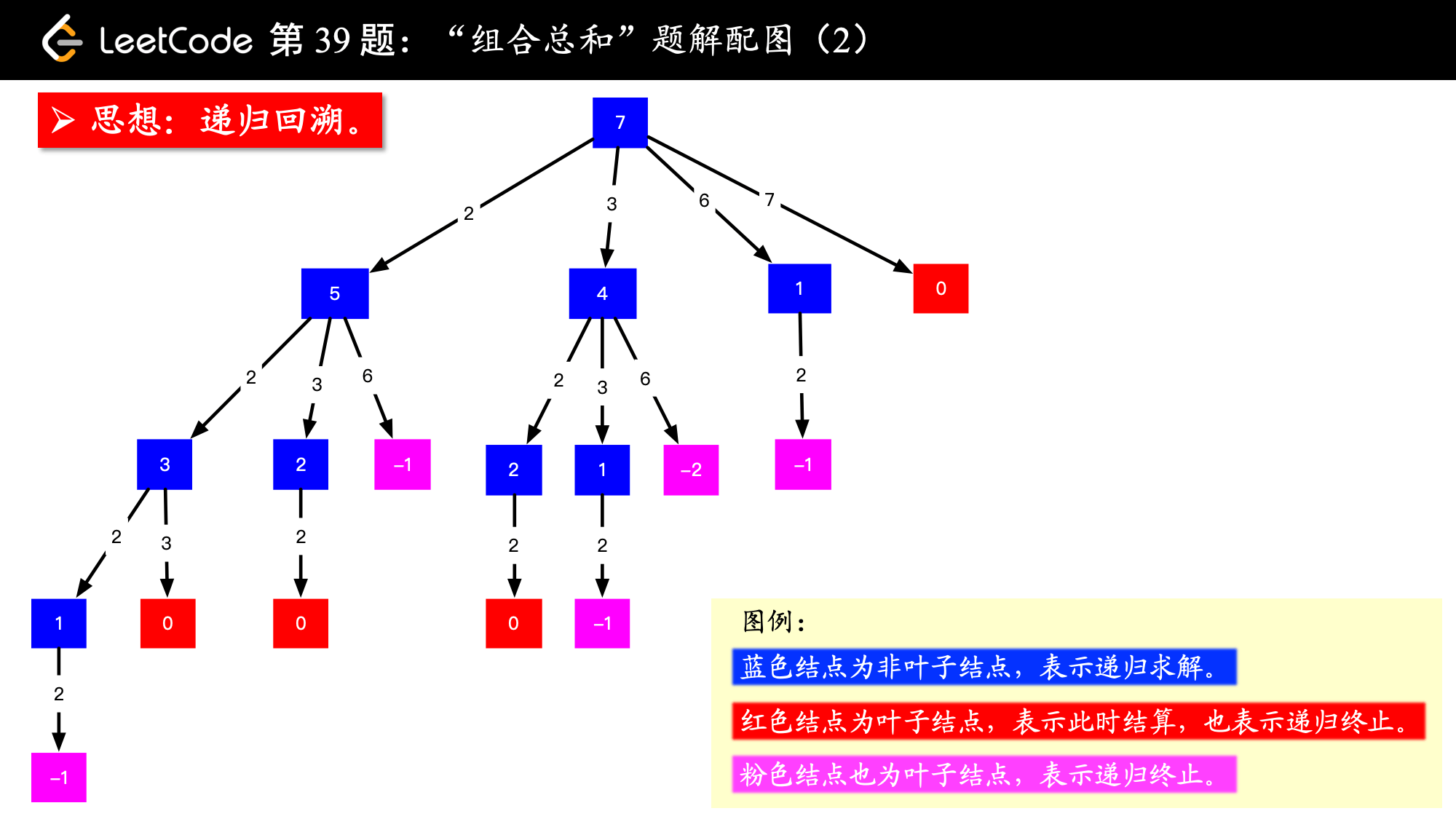
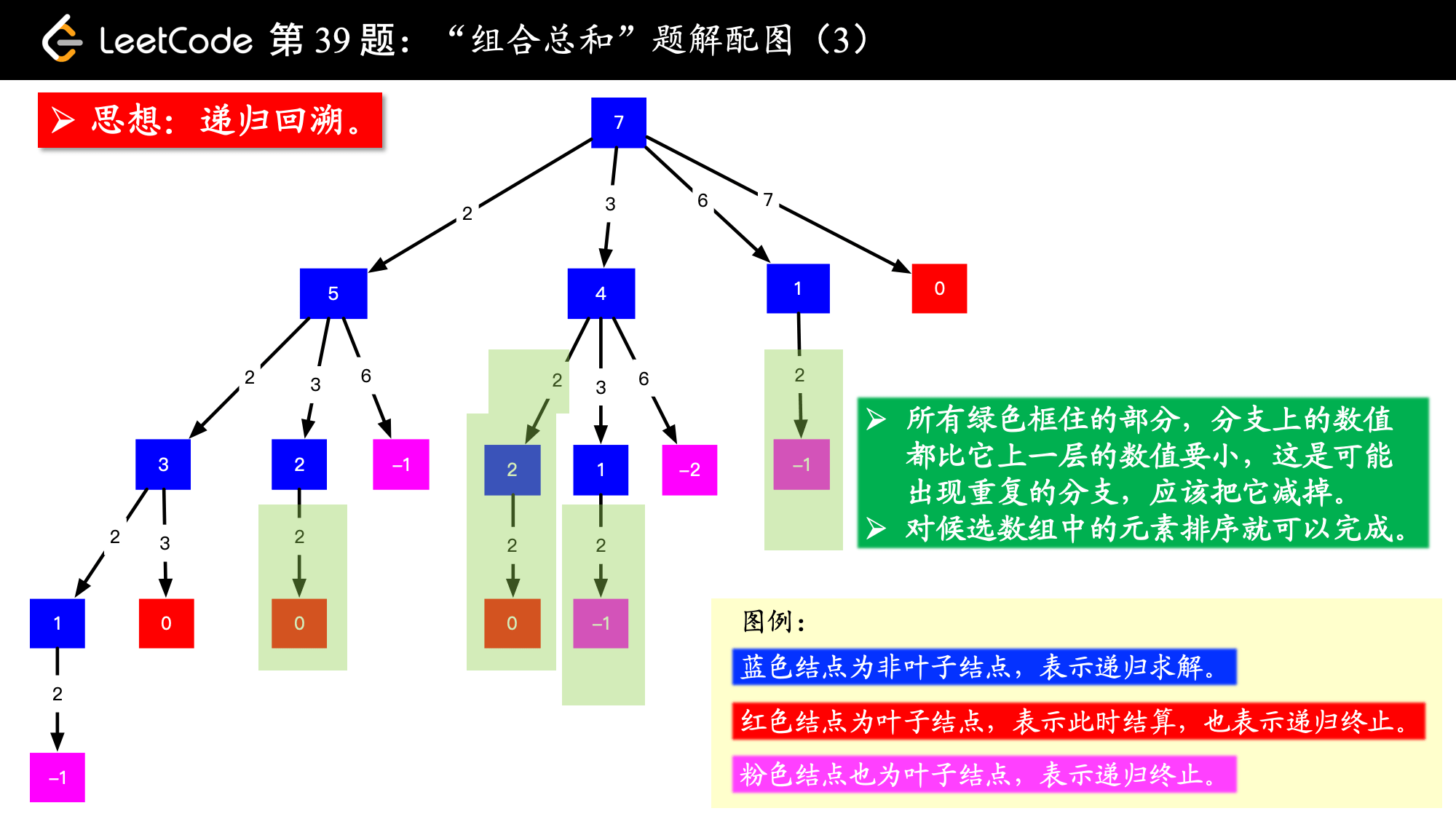
-
一刷
-
二刷
-
三刷
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
/**
* Runtime: 164 ms, faster than 5.05% of Java online submissions for Combination Sum.
* Memory Usage: 45.5 MB, less than 5.19% of Java online submissions for Combination Sum.
* <p>
* ↓
* <p>
* Runtime: 9 ms, faster than 17.31% of Java online submissions for Combination Sum.
* Memory Usage: 41 MB, less than 5.19% of Java online submissions for Combination Sum.
*
* @author D瓜哥 · https://www.diguage.com
* @since 2018-09-16 21:56
*/
public List<List<Integer>> combinationSum(int[] candidates, int target) {
if (null == candidates || candidates.length == 0) {
return Collections.emptyList();
}
Arrays.sort(candidates);
List<List<Integer>> result = new ArrayList<>();
backtrack(candidates, target, result, new ArrayList<>());
return result;
}
private void backtrack(int[] candidates, int target, List<List<Integer>> result, List<Integer> current) {
if (target == 0) {
result.add(new ArrayList<>(current));
}
if (target < 0) {
return;
}
for (int candidate : candidates) {
if (!current.isEmpty() && current.get(current.size() - 1) > candidate) {
continue;
}
current.add(candidate);
backtrack(candidates, target - candidate, result, current);
current.remove(current.size() - 1);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-07-09 19:54:44
*/
public List<List<Integer>> combinationSum(int[] candidates, int target) {
if (candidates == null || candidates.length == 0 || target <= 0) {
return Collections.emptyList();
}
Arrays.sort(candidates);
List<List<Integer>> result = new ArrayList<>();
backtrack(candidates, target, 0, result, new ArrayList<>());
return result;
}
private void backtrack(int[] candidates, int target, int start,
List<List<Integer>> result, List<Integer> perm) {
// 子集和等于 target 时,记录解
if (target == 0) {
result.add(new ArrayList<>(perm));
return;
}
// 剪枝二:从 start 开始遍历,避免生成重复子集
for (int i = start; i < candidates.length; i++) {
int num = candidates[i];
// 剪枝一:若子集和超过 target ,则直接结束循环
if (target < num) {
// 上面排过序,这里直接break
break;
}
perm.add(num);
backtrack(candidates, target - num, i, result, perm);
perm.remove(perm.size() - 1);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
/**
* @author D瓜哥 · https://www.diguage.com
* @since 2024-09-13 22:56:47
*/
public List<List<Integer>> combinationSum(int[] candidates, int target) {
List<List<Integer>> result = new LinkedList<>();
Arrays.sort(candidates);
List<Integer> path = new ArrayList();
backtrack(candidates, target, result, path, 0);
return result;
}
private void backtrack(int[] candidates, int target,
List<List<Integer>> result, List<Integer> path, int index) {
if (target == 0) {
result.add(new ArrayList(path));
return;
}
for (int i = index; i < candidates.length; i++) {
int num = candidates[i];
if (target < num) {
break;
}
path.add(num);
backtrack(candidates, target - num, result, path, i);
path.removeLast();
}
}