友情支持
如果您觉得这个笔记对您有所帮助,看在D瓜哥码这么多字的辛苦上,请友情支持一下,D瓜哥感激不尽,😜
有些打赏的朋友希望可以加个好友,欢迎关注D 瓜哥的微信公众号,这样就可以通过公众号的回复直接给我发信息。
公众号的微信号是: jikerizhi 。因为众所周知的原因,有时图片加载不出来。 如果图片加载不出来可以直接通过搜索微信号来查找我的公众号。 |
567. Permutation in String
Given two strings s1
and s2
, write a function to return true if s2
contains the permutation of s1
. In other words, one of the first string’s permutations is the substring of the second string.
Example 1:
Input: s1 = "ab" s2 = "eidbaooo"
Output: True
Explanation: s2 contains one permutation of s1 ("ba").
Example 2:
Input:s1= "ab" s2 = "eidboaoo"
Output: False
Note:
-
The input strings only contain lower case letters.
-
The length of both given strings is in range [1, 10,000].
解题分析
滑动窗口大法好!
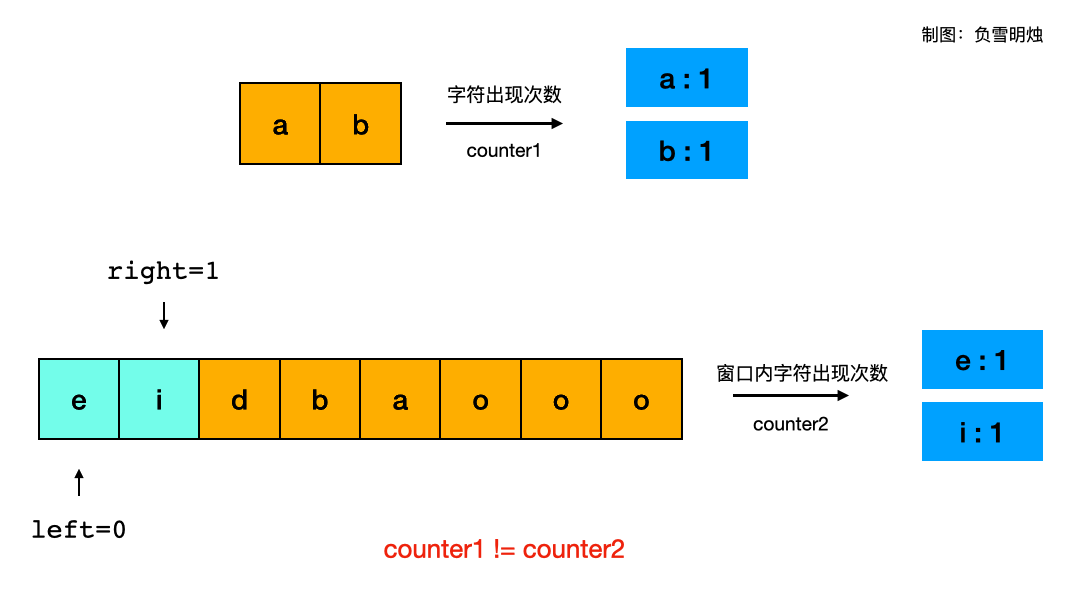
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
/**
* Runtime: 14 ms, faster than 45.41% of Java online submissions for Permutation in String.
* Memory Usage: 39.4 MB, less than 7.69% of Java online submissions for Permutation in String.
*/
public boolean checkInclusion(String s1, String s2) {
if (Objects.isNull(s1) || s1.length() == 0) {
return true;
}
if (Objects.isNull(s2) || s2.length() == 0 || s2.length() < s1.length()) {
return false;
}
Map<Character, Integer> needs = new HashMap<>();
for (char c : s1.toCharArray()) {
needs.put(c, needs.getOrDefault(c, 0) + 1);
}
int left = 0, right = 0;
int match = 0;
Map<Character, Integer> windows = new HashMap<>();
while (right < s2.length()) {
char rChar = s2.charAt(right);
if (needs.containsKey(rChar)) {
int rCount = windows.getOrDefault(rChar, 0) + 1;
windows.put(rChar, rCount);
if (rCount == needs.get(rChar)) {
match++;
}
}
right++;
while (match == needs.size()) {
if (right - left == s1.length()) {
return true;
}
char lChar = s2.charAt(left);
if (needs.containsKey(lChar)) {
int lCount = windows.get(lChar) - 1;
windows.put(lChar, lCount);
if (lCount < needs.get(lChar)) {
match--;
}
}
left++;
}
}
return false;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
/**
* 自己实现
*/
public boolean checkInclusion(String s1, String s2) {
if (s1 == null || s1.isEmpty()
|| s2 == null || s2.isEmpty()
|| s2.length() < s1.length()) {
return false;
}
Map<Character, Integer> need = new HashMap<>();
for (char c : s1.toCharArray()) {
need.put(c, need.getOrDefault(c, 0) + 1);
}
Map<Character, Integer> window = new HashMap<>();
int left = 0, right = 0;
int valid = 0;
while (right < s2.length()) {
char rc = s2.charAt(right);
right++;
if (need.containsKey(rc)) {
window.put(rc, window.getOrDefault(rc, 0) + 1);
if (window.get(rc).equals(need.get(rc))) {
valid++;
}
}
while (need.size() == valid) {
if (right - left == s1.length()) {
return true;
}
char lc = s2.charAt(left);
if (window.containsKey(lc)) {
window.put(lc, window.get(lc) - 1);
if (window.get(lc) < need.get(lc)) {
valid--;
}
}
left++;
}
}
return false;
}